[For Reference only] Authenticate with the legacy OAuth flow
Legacy OAuth flow reference document for Clover apps created before October 2023.
Clover apps must use expiring OAuth tokens generated through the v2/OAuth flow. Note the following:
- Recent applications—Apps created after October 2023 use the v2/OAuth flow to generate expiring tokens (
access_token
andrefresh_token
pair). - Legacy applications—Apps created before October 2023 used the legacy OAuth flow to generate an
auth_token
and must migrate to using expiring tokens (access_token
andrefresh_token
pair) for uninterrupted app functionality.
Watch the tutorial
View | Learn |
---|---|
|
In this video, learn:
|
OAuth in sandbox versus production environment
Our OAuth documentation is created for use with the sandbox environment. To create the OAuth flow for apps on the Clover App Market (in production environments), replacehttps://sandbox.dev.clover.com/
with the correct regional base URL in your requests:
- Europe:
https://eu.clover.com/
- Latin America:
https://la.clover.com/
Steps in the Clover OAuth flow
There are four key steps in the Clover OAuth flow:
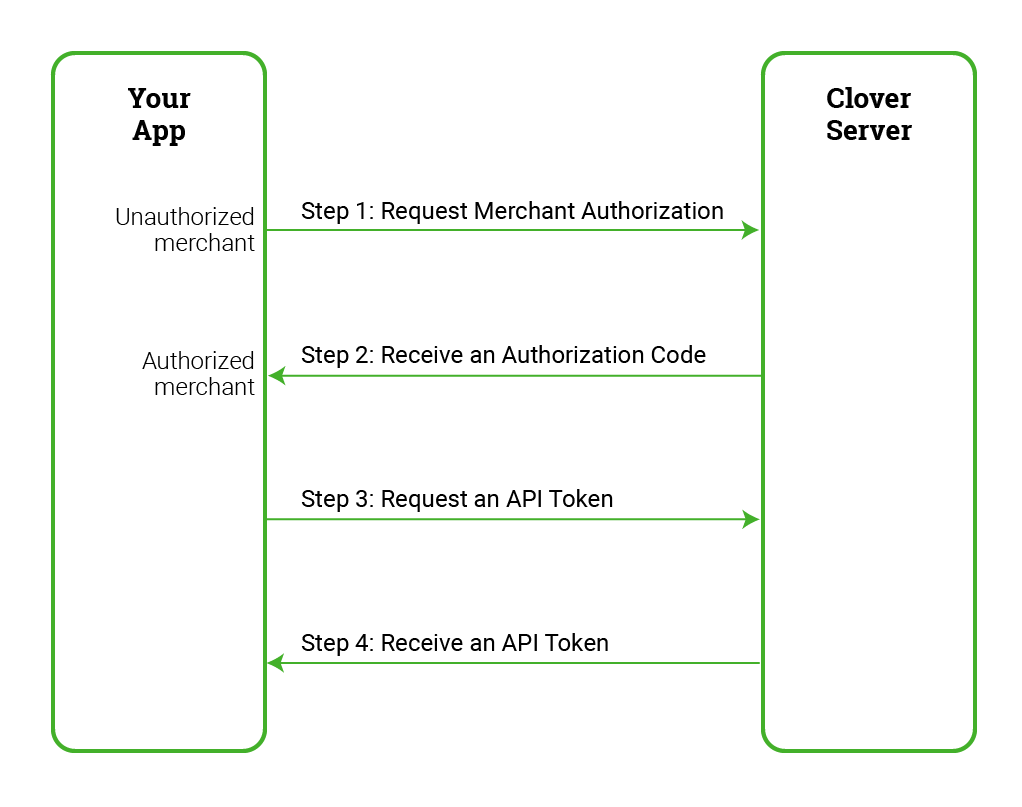
Terminology
To start, let us define a few concepts used in the OAuth flow:
Term | Description |
---|---|
Client ID or App ID | App ID or Client ID that uniquely identifies an app on Clover App Market. This ID is also used to confirm that your app is participating in the OAuth flow. |
Client Secret or App Secret | App Secret or Client Secret is a secret key that Clover assigns to your app. Do not share the Client Secret key publicly. Note: Both the App ID and App Secret are automatically assigned when you create an app. You can view the App ID and App Secret on the App name - App Settings page. Both these values are required for you to authenticate your app with the Clover server and make authorized and authenticated requests to Clover merchant data. |
Merchant ID | Merchant identifier or merchantId that uniquely identifies Clover merchants, including test merchants, on the Clover platform. See Locate the test merchant identifier (merchantId). |
Merchant | Clover merchants can be either unauthorized or authorized to connect to your app. - An unauthorized merchant wants access to your app but has not logged in to their Clover merchant account. Your app redirects this merchant to log in to their merchant account using the following URL format: https://apisandbox.dev.clover.com/oauth/authorize?client_id={APP_ID} - An authorized merchant has logged in to their Clover merchant account. The Clover server redirects the merchant to your app with an authorization code using the following URL format: https://www.example.com/oauth_callback?merchant_id={MERCHANT_ID}&client_id={APP_ID}&code={AUTHORIZATION_CODE} |
Authorization Code | Clover redirects an authorized merchant logged into the Merchant Dashboard to your app along with an authorization code. The Clover server provides this temporary auth_code after the merchant is authenticated. This auth_code is exchanged for an access token during the OAuth flow. |
OAuth or API token | Your app uses the Authorization Code, Client ID, and Client Secret to negotiate with the Clover server for an OAuth API token or access_token . With the API token, your app can make REST API calls and access merchant data. See Use Clover REST API. |
redirect_uri (optional) | After a merchant logs in to their account, you can redirect them to a custom URL using the redirect_uri parameter. If you know the merchant’s account in advance, you can use the merchant_id parameter to skip the account selection step.By default, the Clover server redirects the merchant to your app with an authorization code after they authorize. Receiving this authorization code is a crucial step in the OAuth process for your app on the Clover App Market. |
response_type (optional) | You can build and publish client-based JavaScript and mobile apps on the Clover App Market. For these apps, if you set the response_type parameter as token in the redirect URL, the Clover server sends you an API token and redirects the merchant to your app.Since the API token is publicly accessible, this method is recommended only for merchant-facing apps. To ensure merchants are logging in to a legitimate URL from your app, you can use the state parameter with any string value. See Use the `response_type token method. |
state (optional) | If the Clover server response includes the same state parameter value, it confirms that:1. your app made a legitimate request, and 2. the Clover server has redirected the merchant to your app in response to that legitimate request. |
employee_id (optional) | Uniquely identifies the owner account associated with the merchant business. Send a GET request to v3/merchants/{mId}?expand=owner to identify the merchant business ID and owner account ID. |
Generate an OAuth token
Complete the following steps in the legacy OAuth flow to get an OAuth token.
Prerequisites
Before you can generate an OAuth token, complete the following:
- Set up a sandbox developer account.
- Create an app in the sandbox environment.
- Install your app to your test merchant.
Step 1: Request merchant authorization
The merchant needs to log in to the Clover Merchant Dashboard and install the developer's app from the Clover App Market. By installing the app, the merchant authorizes it to access the merchant's information that the app requires.
If an unauthorized merchant installs your app from the Clover App Market, redirect them to log in to their Clover merchant account and then back to your app, using the following format:
https://apisandbox.dev.clover.com/oauth/authorize?client_id={APP_ID}&redirect_uri={CLIENT_REDIRECT_URL}
If an unauthorized merchant navigates directly to your app URL without installing the app from the Clover App Market:
- Redirect the merchant to log in to their Clover merchant account and then
- Inform the merchant to install your app from the Clover App Market.
Clover provides several parameters to customize the merchant authorization redirect URL. These include: client_id
, merchant_id
, redirect_uri
, response_type
, and state
.
Step 2: Receive an authorization code
Once a merchant is authorized, the Clover server redirects merchants to your app using the Site URL value (under App Settings > REST Configuration) specified in the test Merchant Dashboard.
NOTE
If you have set the
redirect_uri
parameter to a custom location as part of the merchant authorization redirect URL, the server then redirects the merchant to the specified location.
The Clover server redirects the merchant to your app along with the parameters: merchant_id
, client_id
(app_id), employee_id
, and authorization_code
in the following URL format:
https://www.example.com/oauth_callback?merchant_id={MERCHANT_ID}&client_id={APP_ID}&employee_id={EMPLOYEE_ID}&code={AUTHORIZATION_CODE}
With the authorization code, the Clover server confirms that your request for merchant data has been authorized by the merchant. You can use this code to further negotiate with the Clover server for an API token. Every time a merchant is redirected to your app, you can confirm whether the merchant has logged in to their Clover merchant account by checking for an authorization code in the redirect URL.
Step 3: Request and receive an API token
At this stage of the OAuth flow, you exchange your authorization code for an API token. Send an API token request to the Clover server with the parameters: client_id
, client_secret
, and authorization_code
in the following URL format:
https://apisandbox.dev.clover.com/oauth/token?client_id={APP_ID}&client_secret={APP_SECRET}&code={AUTHORIZATION_CODE}
NOTE
Since the API token request consists of sensitive information about your app, this request does not have CORS support. To successfully request an API token, send this request from your app server to the Clover server. When the Clover server responds to the request, retrieve the API token from your app server.
Step 4: Receive an API token
The Clover server responds to your API token request with a JSON object in the following format:
{
"client_id": "{APP_ID}",
"client_secret": "{APP_SECRET}",
"code": "{AUTHORIZATION_CODE}"
}
{
"access_token":"{API_TOKEN}"
}
The API token has a lifespan of one year.
Use the response_type
token method
response_type
token methodWARNING
When you use the
response_type
token method, the API token from the Clover server is publicly accessible. We highly recommend that you use this method only for merchant-facing apps.
All your apps on the Clover App Market follow the OAuth flow to retrieve an API token from the Clover server. You can also build and publish client-based JavaScript and mobile apps on the Clover App Market. For these client-based apps, Clover provides you with the response_type
token method to retrieve an API token as soon as the merchant is authorized.
To use the response_type
token method:
- Log in to your Developer Dashboard.
- From the left navigation menu, click Your Apps > App name > App Settings. The App name - App Settings page lets you view and configure settings and permissions that your app requires to access Clover merchant data.
- Select Web app on the App Type page. See Select App Type.
The REST Configuration displays on the App Settings page. - Click REST Configuration. The Edit REST Configuration page appears.
- In the Default OAuth Response field, select Token (Testing Only).
- In the merchant authorization redirect URL (step 1 of the OAuth flow), set the value of the
response_type
parameter astoken
.
The Clover server directly sends you an API token and redirects the authorized merchant to your app using the following URL format:
https://example.com/javascript_app?&merchant_id={MERCHANT_ID}&client_id={APP_ID}&employee_id={EMPLOYEE_ID}#access_token={API_TOKEN}
Updated 18 days ago