Issue a manual refund
United States
Use the CreditRequestIntentBuilder
class to build an Intent to initiate an activity to create a manual refund. Manual refund is not associated with an existing payment. See manual refund for more information.
Prerequisites
- Read overview of the Clover platform.
- Create a global developer account with a default test merchant account.
- Order a Clover Developer Kit (Dev Kit) and set it up.
- Use the required Android SDK versions.
Steps
- Build a manual refund request.
val context = this
val externalPaymentId = "<posPaymentId>" // should be unique for each request
val amount = 1000L
val builder = CreditRequestIntentBuilder(externalPaymentId, amount)
val creditRequestIntent = builder.build(context)
Context context = this;
String externalPaymentId = "<posPaymentId>"; // should be unique for each request
Long amount = 1000L;
CreditRequestIntentBuilder builder = new CreditRequestIntentBuilder(externalPaymentId, amount);
Intent creditRequestIntent = builder.build(context);
-
Use the Intent to initiate the activity to process the manual refund request.
-
Use the optional
CardOptions
andReceiptOptions
classes on the builder to provide additional processing instructions.
CardOptions
The CardOptions
class provides a static method to set the supported card entry methods and a cardNotPresent
flag.
Method | Description |
---|---|
Instance( cardEntryMethods : Set<CardEntryMethod>, cardNotPresent : Boolean) | Sets card entry flags to create a CardOptions instance.Note: When no value or the null value is passed in the instance, the system takes the merchant's default setting. |
Example—Set the CardOptions to All
val externalPaymentId = "<posPaymentId>" // should be unique for each request
val amount = 1000L
val builder = CreditRequestIntentBuilder(externalPaymentId, amount)
val cardOptions =
CreditRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.All(), null)
val creditRequestIntent = builder.cardOptions(cardOptions).build(context)
String externalPaymentId = "<posPaymentId>"; // should be unique for each request
Long amount = 1000L;
CreditRequestIntentBuilder builder = new CreditRequestIntentBuilder(externalPaymentId, amount);
CreditRequestIntentBuilder.CardOptions cardOptions =
CreditRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.All(), null);
Intent creditRequestIntent = builder.cardOptions(cardOptions).build(context);
ReceiptOptions
The ReceiptOptions
class provides a static helper method to simplify the creation of ReceiptOptions
.
Method | Description |
---|---|
Default(cloverShouldHandleReceipts: Boolean) | Indicates whether Clover is handling the printing or sending of SMS and email receipts. |
SkipReceiptSelection() | Skips the selection and display of a receipt. For the No Receipt option, the receipt selection step is skipped, and no receipt is printed. |
Instance ( {{cloverShouldHandleReceipts : Boolean, }} {{smsReceiptOption : SmsReceiptOption, }} {{emailReceiptOption : EmailReceiptOption, }} {{printReceiptOption : PrintReceiptOption, }} noReceiptOption : NoReceiptOption) | Activates or deactivates the receipt selection button on the receipt screen. Indicates whether Clover is handling the printing or sending of SMS and email receipts. |
Example—Deactivate SMS and email receipt buttons and set cloverShouldHandleReceipts to true.
val externalPaymentId = "<posPaymentId>" // should be unique for each request
val amount = 1000L
val builder = CreditRequestIntentBuilder(externalPaymentId, amount)
val receiptOptions = CreditRequestIntentBuilder.ReceiptOptions.Instance(true, CreditRequestIntentBuilder.ReceiptOptions.SmsReceiptOption.Disable(), CreditRequestIntentBuilder.ReceiptOptions.EmailReceiptOption.Disable(), CreditRequestIntentBuilder.ReceiptOptions.PrintReceiptOption.Enable(), CreditRequestIntentBuilder.ReceiptOptions.NoReceiptOption.Enable())
val creditRequestIntent = builder.receiptOptions(receiptOptions).build(context)
String externalPaymentId = "<posPaymentId>"; // should be unique for each request
Long amount = 1000L;
CreditRequestIntentBuilder builder = new CreditRequestIntentBuilder(externalPaymentId, amount);
CreditRequestIntentBuilder.ReceiptOptions receiptOptions = CreditRequestIntentBuilder.ReceiptOptions.Instance(
true,
CreditRequestIntentBuilder.ReceiptOptions.SmsReceiptOption.Disable(),
CreditRequestIntentBuilder.ReceiptOptions.EmailReceiptOption.Disable(),
CreditRequestIntentBuilder.ReceiptOptions.PrintReceiptOption.Enable(),
CreditRequestIntentBuilder.ReceiptOptions.NoReceiptOption.Enable());
Intent creditRequestIntent = builder.receiptOptions(receiptOptions).build(context);
Result on the device
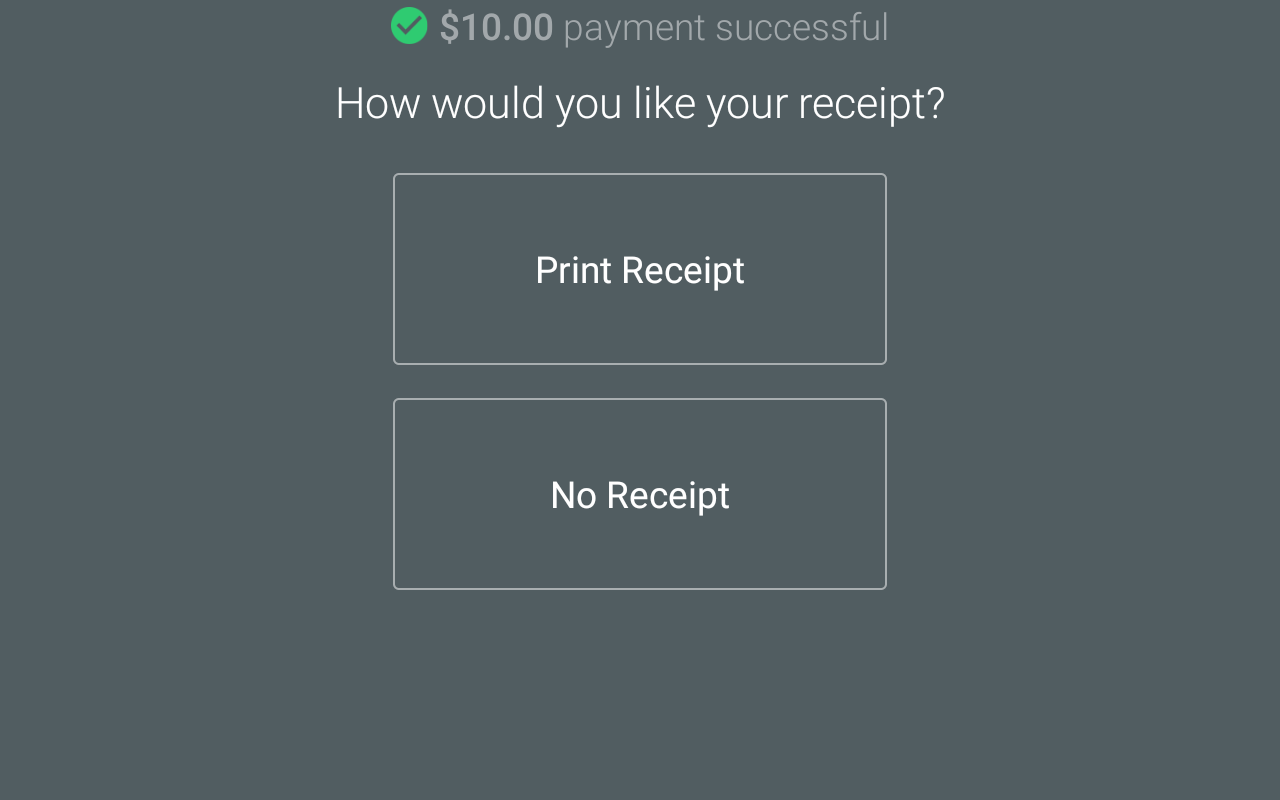
Manual refund: Receipt screen
Additional fields
Additional fields for theCreditRequestIntentBuilder
classes are:
Field | Description |
---|---|
externalPaymentId: String | Custom external payment ID persists with the transaction. |
amount: Long | Total credit amount. |
Response details
- When the credit flow completes, the response is available through
onActivityResult()
. - For a successful response, retrieve the following:
- Credit object using the Intent:
CreditRequestIntentBuilder.Response.CREDIT
- Selected receipt option using the Intent:
Intents.EXTRA_RECEIPT_DELIVERY_TYPE
- Status whether the receipt option was processed or requested, using the Intent:
Intents.EXTRA_RECEIPT_DELIVERY_STATUS
- Email or SMS value, if entered, using the Intent:
CreditRequestIntentBuilder.Response.ENTERED_RECEIPT_VALUE
- Credit object using the Intent:
- For an unsuccessful response, retrieve the failure message using the Intent:
CreditRequestIntentBuilder.Response.FAILURE_MESSAGE
Response example
fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == CREDIT_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
val credit: Credit = data?.getParcelableExtra(CreditRequestIntentBuilder.Response.CREDIT)
val receiptDeliveryType = data?.getStringExtra(CreditRequestIntentBuilder.Response.RECEIPT_DELIVERY_TYPE)
val receiptDeliveryStatus = data?.getStringExtra(CreditRequestIntentBuilder.Response.RECEIPT_DELIVERY_STATUS)
val enteredReceiptValue = data?.getStringExtra(CreditRequestIntentBuilder.Response.ENTERED_RECEIPT_VALUE)
} else {
// credit failed, check for error
val failureMessage = data?.getStringExtra(CreditRequestIntentBuilder.Response.FAILURE_MESSAGE)
}
}
}
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == CREDIT_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
Credit credit = data.getParcelableExtra(CreditRequestIntentBuilder.Response.CREDIT);
String receiptDeliveryType = data.getStringExtra(CreditRequestIntentBuilder.Response.RECEIPT_DELIVERY_TYPE);
String receiptDeliveryStatus = data.getStringExtra(CreditRequestIntentBuilder.Response.RECEIPT_DELIVERY_STATUS);
String enteredReceiptValue = data.getStringExtra(CreditRequestIntentBuilder.Response.ENTERED_RECEIPT_VALUE);
} else {
// credit failed, check for error
String failureMessage = data.getStringExtra(CreditRequestIntentBuilder.Response.FAILURE_MESSAGE);
}
}
}
Updated 2 months ago