Tokenize a card
Use theTokenizeCardRequestIntentBuilder
class build an Intent to tokenize a card for Clover Ecommerce endpoints.
Prerequisites
- Read overview of the Clover platform.
- Create a global developer account with a default test merchant account.
- Order a Clover Developer Kit (Dev Kit) and set it up.
- Use the required Android SDK versions.
- Core Payments App (CPA) installed on the Clover Gen 3 devices to use the card tokenization feature. If your device does not have CPA installed, contact Clover support to use this feature.
Build a tokenize request
Build a tokenize card request.
val context = this
val builder = TokenizeCardRequestIntentBuilder()
val intent = builder.build(context)
Context context = this;
TokenizeCardRequestIntentBuilder builder = new TokenizeCardRequestIntentBuilder();
Intent intent = builder.build(context);
CardOptions
The CardOptions
class provides a static method to set the supported card entry methods.
Method | Description |
---|---|
Instance(cardEntryMethods : Set<CardEntryMethod>) | Set the cardEntryMethods flag to create a CardOptions instance.You can limit the card entry methods—for example, CardReaders(), Manual(), All(). |
Example—Set the card entry method to CardReaders
val builder = TokenizeCardRequestIntentBuilder()
val cardOptions = TokenizeCardRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.CardReaders())
val intent = builder.cardOptions(cardOptions).build(context)
TokenizeCardRequestIntentBuilder builder = new TokenizeCardRequestIntentBuilder();
TokenizeCardRequestIntentBuilder.CardOptions cardOptions = TokenizeCardRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.CardReaders());
Intent intent = builder.cardOptions(cardOptions).build(context);
Additional fields
Field | Description |
---|---|
suppressConfirmation:Boolean? | Suppress—or skip—the customer's card information confirmation pop-up that asks customers if the merchant can store their information. |
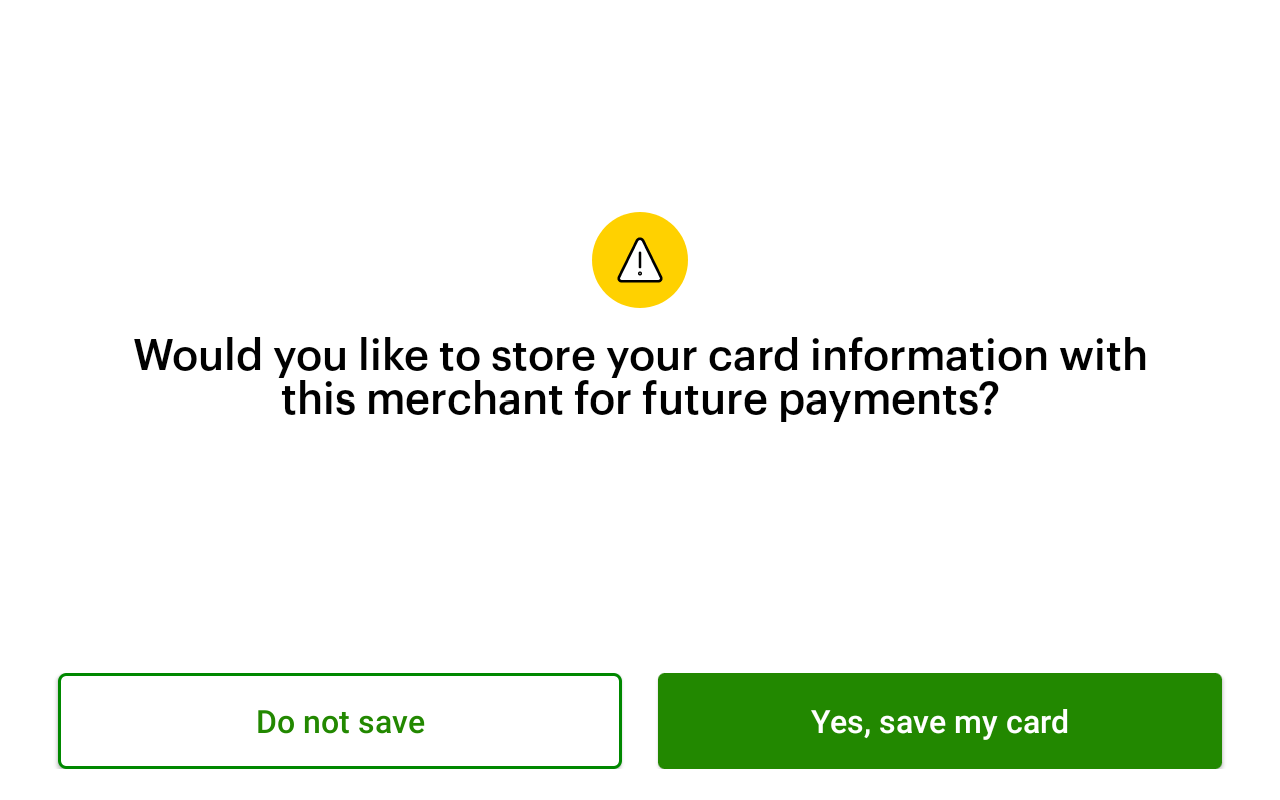
Token confirmation pop-up
Response details
When the tokenized card flow completes, the response is available through onActivityResult()
.
For a successful request:
- Retrieve the
token
object using the Intent:TokenizeCardRequestIntentBuilder.Response.TOKEN
- Retrieve the
tokenType
object using the Intent:TokenizeCardRequestIntentBuilder.Response.TOKEN_TYPE
- Retrieve the
suppressConfirmation
status using the Intent:TokenizeCardRequestIntentBuilder.Response.SUPPRESS_CONFIRMATION
- Retrieve the
card
object using the Intent:TokenizeCardRequestIntentBuilder.Response.CARD
For an unsuccessful request, retrieve the failure message using the Intent: TokenizeCardRequestIntentBuilder.Response.FAILURE_MESSAGE
Response example
fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == TOKENIZE_CARD_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
val token = data.getStringExtra(Intents.EXTRA_TOKEN)
val tokenType = data.getStringExtra(Intents.EXTRA_TOKEN_TYPE)
val suppressConfirmation = data.getBooleanExtra(Intents.EXTRA_SUPPRESS_CONFIRMATION, false)
val card:Card = data.getParcelableExtra(Intents.EXTRA_CARD)
} else {
// tokenize failed, check for error
val failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE)
}
}
}
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == TOKENIZE_CARD_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
String token = data.getStringExtra(Intents.EXTRA_TOKEN);
String tokenType = data.getStringExtra(Intents.EXTRA_TOKEN_TYPE);
boolean suppressConfirmation = data.getBooleanExtra(Intents.EXTRA_SUPPRESS_CONFIRMATION, false);
Card card = data.getParcelableExtra(Intents.EXTRA_CARD);
} else {
// tokenize failed, check for error
String failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE);
}
}
}
Additional requirements for developers
If your application stores tokens for subsequent transactions, you are responsible for complying with card-on-file (COF) mandates, also known as the Stored Credentials Transaction framework.
Clover collects a general consent agreement from the customer to proceed with either a card tokenization request and/or payment. Card-on-file rules require additional steps that merchants and software developers must adhere to compliance. Merchants are still responsible for the following activities when saving customer cards (tokens) on file:
- Inform the account issuer that payment credentials are now stored on file. To do this either process an initial payment or complete a $0 account verification through the Clover REST Pay Display API.
- Disclose to cardholders how the credentials are used.
- Notify cardholders when any changes are made to the terms of use.
- For more information about safekeeping tokenized card data, see our blog Ecommerce Tokenization: Understanding Methods that Keep Card Data Safe.
Updated 3 months ago