Adjust a tip
United States
Use the TipAdjustRequestIntentBuilder
class to build an Intent to add a tip to a payment.
This will NOT prompt the customer for a tip; rather, it allows a merchant to apply a tip to an existing payment, for example, from a tip amount left on a receipt. A UI will be displayed if the tip amount is not provided (null), allowing a merchant to enter a tip amount.
Prerequisites
- Read overview of the Clover platform.
- Create a global developer account with a default test merchant account.
- Order a Clover Developer Kit (Dev Kit) and set it up.
- Use the required Android SDK versions.
Request a headless tip adjust
Build a tip adjust request with tipAmount
that is not entered through a user interface.
val paymentId = payment.id // should be id from a payment
val tipAmount = 300L
val builder = TipAdjustRequestIntentBuilder.AdjustTip(paymentId, tipAmount)
val intent = builder.build(context)
String paymentId = payment.getId(); // should be id from a payment
long tipAmount = 300l;
TipAdjustRequestIntentBuilder builder = TipAdjustRequestIntentBuilder.AdjustTip(paymentId, tipAmount);
Intent intent = builder.build(context);
Request a tip adjust with keypad
Build a tip adjust request with notipAmount
.
val paymentId = payment.id // should be id from a payment
val builder = TipAdjustRequestIntentBuilder.AdjustTip(paymentId, null)
val intent = builder.build(context)
String paymentId = payment.getId(); // should be id from a payment
TipAdjustRequestIntentBuilder builder = TipAdjustRequestIntentBuilder.AdjustTip(paymentId, null);
Intent intent = builder.build(context);
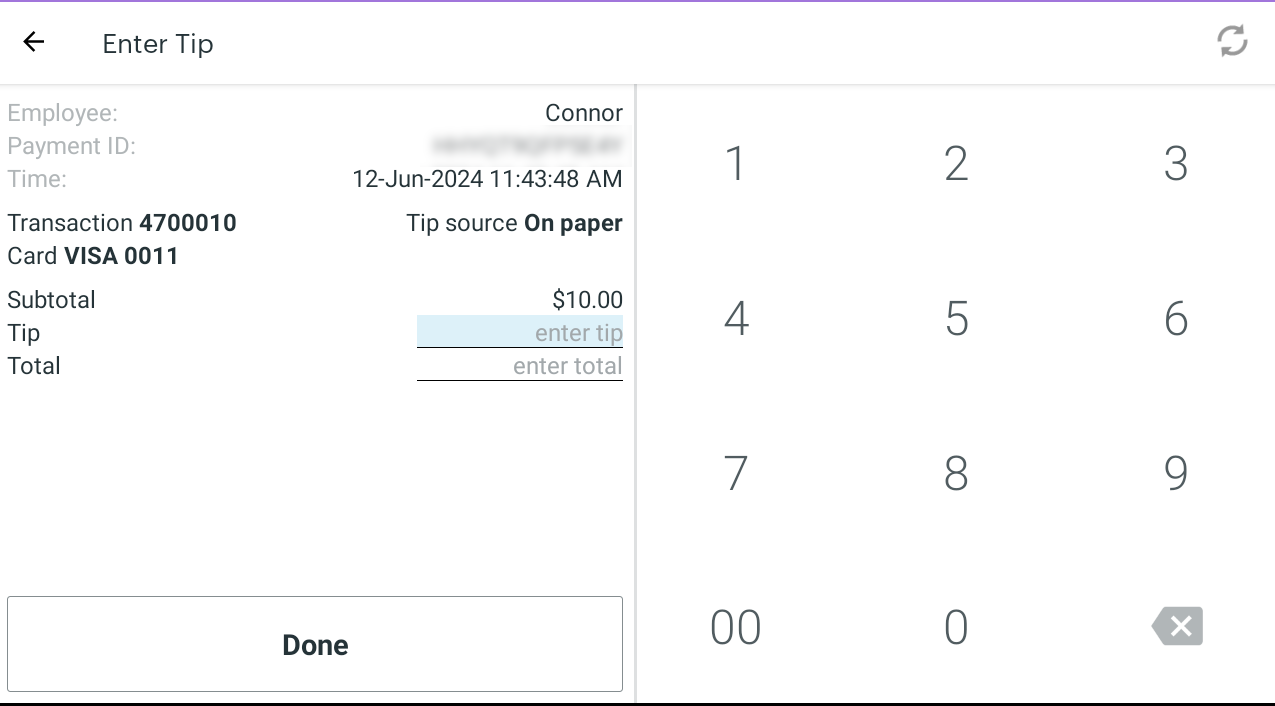
Tip adjust keypad page: no tipAmount
entered
Response details
When the tip adjust flow completes, the response is available through onActivityResult()
.
Check the TipAdjustRequestIntentBuilder.Response
class for the response fields.
- For a successful request, retrieve the mapping of
paymentId
to selectedtipAmount
values using the Intent:TipAdjustRequestIntentBuilder.Response.TIP_AMOUNTS
- For an unsuccessful request (error, failure, or cancellation), retrieve the optional failure message using the Intent:
TipAdjustRequestIntentBuilder.Response.FAILURE_MESSAGE
Response example
fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == TIP_ADJUST_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
val tipAmounts: Map<String, Long> = data?.getSerializableExtra(TipAdjustRequestIntentBuilder.Response.TIP_AMOUNTS) as Map<String, Long>
} else {
// tip adjust request failed, check for error
val failureMessage = data?.getStringExtra(TipAdjustRequestIntentBuilder.Response.FAILURE_MESSAGE)
}
}
}
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == TIP_ADJUST_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
Map<String, Long> tipAmount = data.getSerializableExtra(TipAdjustRequestIntentBuilder.Response.TIP_AMOUNTS);
} else {
// tip adjust request failed, check for error
String failureMessage = data.getStringExtra(TipAdjustRequestIntentBuilder.Response.FAILURE_MESSAGE);
}
}
}
Updated 4 months ago