iOS—Partial OAuth implementation
Overview
In the iOS—Partial OAuth implementation, you perform the OAuth login flow within your app while the SDK manages the refresh tokens. Use Partial OAuth when you want to control the login flow but let the SDK handle token refresh. This implementation offers more control over the login experience but requires additional development effort to handle the OAuth flow within your app.
Pros:
- Automatic token management—The SDK manages refresh tokens, ensuring you always have a valid token.
- Customizable login screen—You can display the Clover login screen directly in your app using your preferred brand design.
- Seamless user experience—You can implement the OAuth login flow without exiting the app, providing a smoother user experience.
Cons:
- Custom web view implementation—You need to write your own web view implementation to execute the OAuth login flow.
- Code response handling—You need to monitor and intercept the code response from the OAuth login flow within your app.
- Increased complexity—Significantly more complicated implementation in your code than the Full OAuth flow.
Before you begin
If your mobile app is running on iOS and consuming the CloverPaymentSDK, you need to configure your mobile app for OAuth. Clover uses OAuth 2.0 to authenticate the users of your app to Clover servers. Before you begin, review the OAuth flow and terminology.
To implement the OAuth flow, you need to:
- Create a Clover app and install it on your test merchant to enable OAuth. The Clover app has an associated App ID and App Secret that Clover transfers to the iOS app to give it permission to perform OAuth.
- Use Associated Domains in the iOS app and an
apple-app-site-association
file on a server you control to enable OAuth callbacks from Clover login servers to your app.
For detailed information, see iOS—Clover Go SDK quick start guide.
Prerequisites
- Create a global developer account with a default test merchant account.
- Order a Clover Go reader Developer Kit (Dev Kit) and set it up.
- Use an iOS Device (iOS 14+).
- Use an iOS Developer Account.
- Use Xcode 14 or higher.
- Install CocoaPods.
- Install CloverPayments SDK from CocoaPods.
- Charge Clover Go reader—Device battery charging requirement. Several operations on your Clover Go reader require at least 30% battery. Charge your device before you configure your iOS project using the instructions in this guide.
Steps
First launch
- In your app, implement the OAuth login flow:
- Use the Low-trust apps—Auth code flow with PKCE for mobile apps where the app secret cannot be kept secret due to the nature of distributed app binaries.
Note: Use theCloverPaymentSDK.OAuthCodeChallenge
object to facilitate the PKCE flow by generating the verifier and challenge objects for use in the flow. - Get the code response and pass that information to the SDK.
- Use the Low-trust apps—Auth code flow with PKCE for mobile apps where the app secret cannot be kept secret due to the nature of distributed app binaries.
- Initialize the SDK by calling
CloverPaymentSDK.shared.setup
and passing in your configuration object.- Include a
CloverPaymentSDK.PartialOAuth
object in the configuration. - In the
PartialOAuth
object, include the auth code received from the OAuth login flow and the Challenge information used with the PKCE flow.
- Include a
The CloverPaymentSDK exchanges the code for a token, stores the token in Keychain for the next launch, and then calls your token change callback registered in step 1.
Subsequent launches
Initialize the SDK by calling CloverPaymentSDK.shared.setup
and pass your configuration object.
- Include a
CloverPaymentSDK.PartialOAuth
object in the configuration. - In the
PartialOAuth
object set the code to nil to signal to the SDK to use the previously stored token.
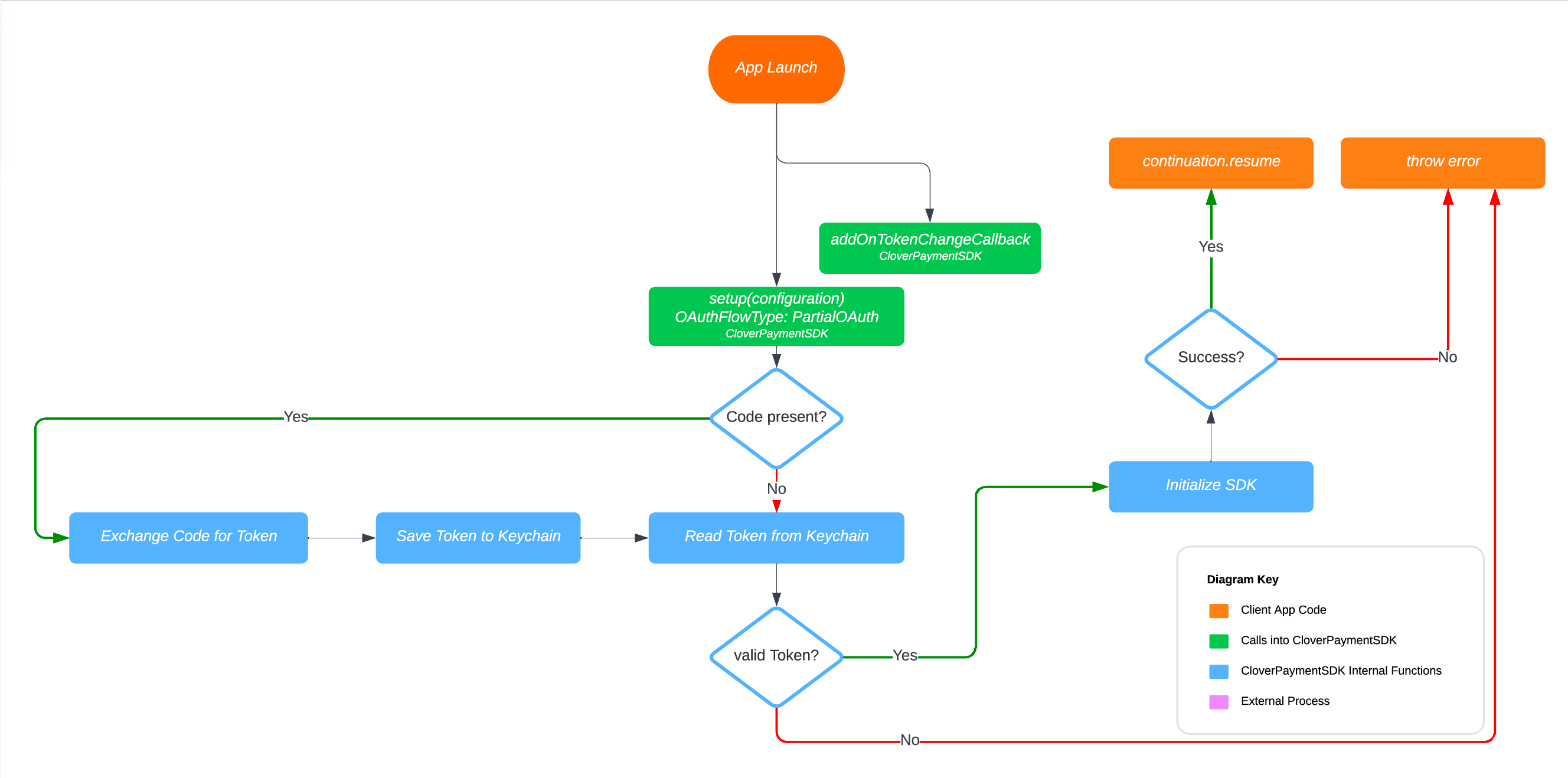
iOS—Partial OAuth
Related topics
Updated 7 months ago