(MSC Pilot) Manage service charges for Web apps
Learn how to use the MSC REST API to manage multiple service charges (MSC) for Web apps.
This topic describes how to use the MSC REST API to manage multiple service charges (MSC) for Web apps. The endpoints are organized into two sections for merchant-level and order-level endpoints:
REST APIs to manage service charges | Description |
---|---|
Merchant-level REST API endpoints | Use to: - Get a list of all service charges - Get details for single service charge |
Order-level REST API endpoints | Use to: - Add one or more service charges associated with a merchant to a given order - Remove one or more service charges associated with a merchant from an order - Get a single service charge associated with an order - Get the list of service charges associated with a given ord |
NOTE
To access the complete, testable MSC REST API reference endpoints, see the MSC REST API Index.
Merchant-level REST API endpoints
Get service charges associated with a merchant
Use the v3/merchants/{mId}/order_fees
endpoint to:
- get a list of all service charges
- get a single charge associated with a merchant
Get a list of all service charges
- Send a GET request to
v3/merchants/{mId}/order_fees
. - Enter the merchant identifier (
mId
) in the request.
curl --request GET \
--url 'https://sandbox.dev.clover.com/v3/merchants/{mId}/order_fees' \
--header 'content-type: application/json' \
--header 'Authorization: Bearer {access_token}'
{
"elements": [
{
"id": "DPDDK16CGET6E",
"name": "StaffMed",
"percentage": 1000,
"createdTime": 1705681517000,
"modifiedTime": 1705681517000
},
{
"id": "7QC732JVN2DXP",
"name": "Delivery",
"percentage": 40000,
"createdTime": 1705029883000,
"modifiedTime": 1705029883000,
"type": ""
},
{
"id": "HHBECXV21DRQ4",
"name": "LargeParty",
"percentage": 30000,
"createdTime": 1704480354000,
"modifiedTime": 1705360002000,
"type": ""
}
],
"href": "/v3/merchants/HDHEQBN4ARCF5/order_fees"
}
Request parameters
Property | Type | Description | Required/ Optional |
---|---|---|---|
mId | string | Merchant identifier. | Required |
Query filter | string | Filter fields: - modifiedTime - amount - serviceChargeUuid - type - percentage - name - createdTime - id - deletedTime | Required |
Response parameters
Property | Type | Description |
---|---|---|
id | string | Service charge identifier. |
name | string | Service charge name. |
percentage | integer | Service charge amount in percent. |
createdTime | integer | Timestamp when the order fee was created. |
modifiedTime | integer | Timestamp when the service charge was last modified. |
Get details for a single service charge
- Send a GET request to
v3/merchants/{mId}/order_fees/{orderFeeId}
. - Enter the merchant identifier (
mId
) and the order fee identifier (orderFeeId
) in the request.
curl --request GET \
--url 'https://apisandbox.dev.clover.com/v3/merchants/{mId}/order_fees/{orderFeeId}' \
--header 'content-type: application/json' \
--header 'Authorization: Bearer {access_token}'
{
"id": "HHBECXV21DRQ4",
"name": "LargeParty",
"percentage": 30000,
"createdTime": 1704480354000,
"modifiedTime": 1705360002000,
"type": ""
}
Request parameters
Property | Type | Description | Required/ Optional |
---|---|---|---|
mId | string | Merchant identifier. | Required |
orderFeeId | string | Order fee identifier. | Required |
Response parameters
Property | Type | Description |
---|---|---|
id | string | Order fee identifier. |
name | string | Service charge (order fee) name. |
percentage | integer | Service charge amount in percent. |
createdTime | integer | Time when the service charge was recorded on the server. |
modifiedTime | integer | Time when the service charge was modified on the server. |
Order-level REST API endpoints
Use the isOrderFee
object flag to control where service charges appear
isOrderFee
object flag to control where service charges appearAt the API level, service charges are handled as line items (of service charge type). However, in your app interface, service charges should not display with the line items—they should be listed after the subtotal because taxes are not applied to service fees.
You can control the placement of service charges in your app interface using the isOrderFee
object flag.
- For regular (non-service charge) lineItems the
LineItem
object flag is set toisOrderFee
: false. - When using
orderFeeLineItem
, set theLineItem
object flag toisOrderFee
: true.
Make sure the response for the service charges found indicates isOrderFee
: true.
For example:
{
"id": "S4XK3VG1RXK1C",
"orderRef": {
"id": "P6JVBY8V8KFEC"
},
"name": "test order fee 2",
"price": 0,
"note": "test note",
"printed": false,
"createdTime": 1709732377000,
"orderClientCreatedTime": 1709732166000,
"exchanged": false,
"refunded": false,
"isRevenue": false,
"percentage": 190000,
"orderFee": {
"id": "7M8463224GJ5R"
},
"isOrderFee": true
}
Make service charges display in the correct location
- For the regular order line items, filter out service charge line items using the
isOrderFee
: false object flag. - For the post-subtotal item list, include service charges using the
isOrderFee
: true object flag.
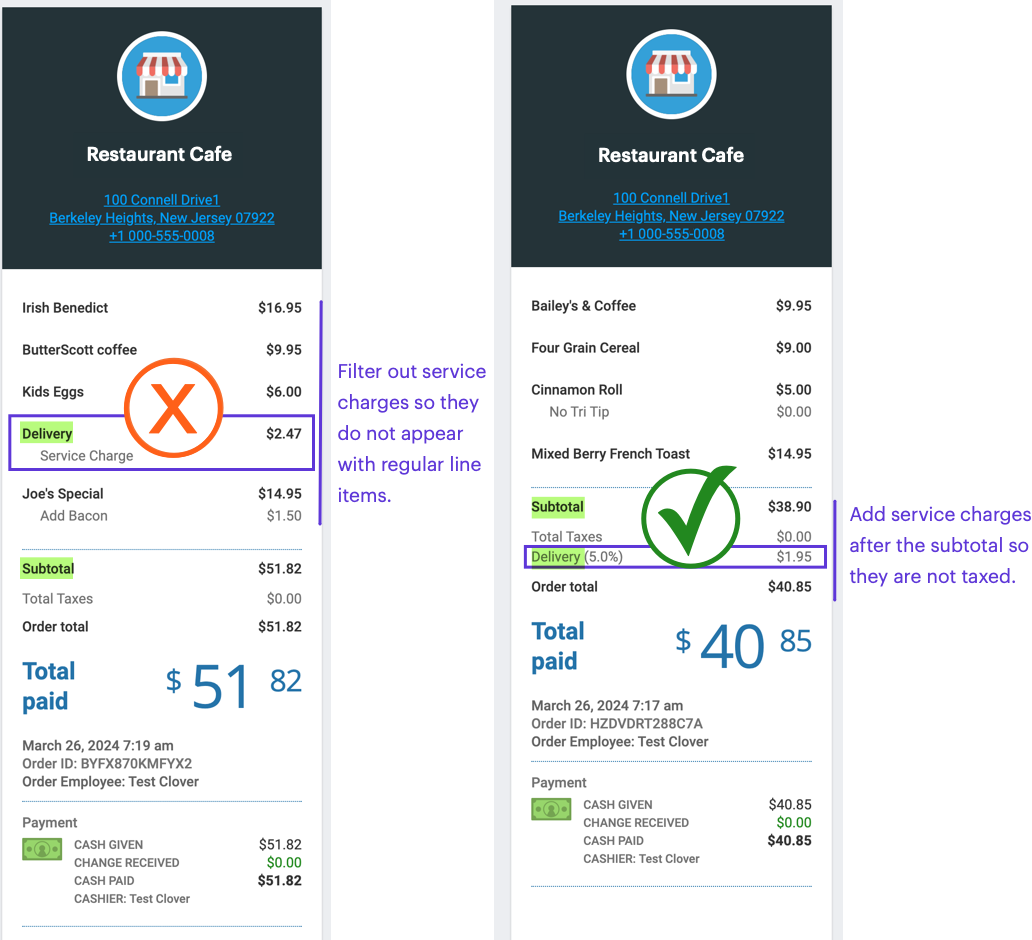
Illustration of correct and incorrect placement of service charges in an order receipt
Get a list of service charges associated with an order
- Send a GET request to the
v3/merchants/{mId}/orders/{orderId}/order_fee_line_items
endpoint. - Enter the merchant identifier (
mId
) and the order identifier (orderId
) in the request.
curl --request GET \
--url 'https://apisandbox.dev.clover.com/v3/merchants/{mId}/orders/{orderId}/order_fee_line_items' \
--header 'content-type: application/json' \
--header 'Authorization: Bearer {access_token}'
{
"elements": [
{
"id": "S4XK3VG1RXK1C",
"orderRef": {
"id": "P6JVBY8V8KFEC"
},
"name": "order fee",
"price": 10,
"note": "test note",
"printed": false,
"createdTime": 1709732377000,
"orderClientCreatedTime": 1709732166000,
"exchanged": false,
"refunded": false,
"isRevenue": false,
"percentage": 190000,
"orderFee": {
"id": "7M8463224GJ5R"
},
"isOrderFee": true
}
],
"href": "http://sandbox.dev.clover.com/v3/merchants/{mid}/orders/{orderId}/order_fee_line_items?limit=100"
}
Request parameters
Property | Type | Description | Required/ Optional |
---|---|---|---|
mId | string | Merchant identifier. | Required |
orderId | string | Order identifier. | Required |
Expand query | string | Expandable fields: - employee - order type - payments | Optional |
Response parameters
Parameter | Type | Description |
---|---|---|
id | string | Merchant identifier. |
orderRefid | string | Reference identifier of the order fee with which the service charge is associated. |
name | string | Name of the order fee. |
price | integer | Order fee price. |
note | string | Comments or information, if any. |
createdTime | integer | Time when the service charge was recorded on the server. |
orderClientCreatedTime | integer | Order client-created time for user data of line item. |
percentage | integer | Service charge amount in percent. |
orderFeeid | string | Order fee identifier. |
Add service charges associated with a merchant to an order
NOTE
No tax on order fees—Since MSC order fees are treated as line items, and order fees are non-taxed items, you must mark each order fee line item as a 0% No Tax Applied tax.
For more information, see Tax report example 5: zero tax.
To add one or more service charges associated with a merchant to an order:
- Send a POST request to
/v3/merchants/{mId}/orders/{orderId}/order_fee_line_items
. - Enter the merchant identifier (
mId
) and order identifier (orderId
) in the request.
curl --request POST \
--url 'https://apisandbox.dev.clover.com/v3/merchants/{mId}/orders/{orderId}/order_fee_line_items'/
--header 'content-type: application/json' \
--header 'Authorization: Bearer {access_token}'
{
"id": "S4XK3VG1RXK1C",
"orderRef": {
"id": "P6JVBY8V8KFEC"
},
"name": "test order fee 2",
"price": 0,
"note": "test note",
"printed": false,
"createdTime": 1709732377000,
"orderClientCreatedTime": 1709732166000,
"exchanged": false,
"refunded": false,
"isRevenue": false,
"percentage": 190000,
"orderFee": {
"id": "7M8463224GJ5R"
},
"isOrderFee": true
}
}
Request parameters
Parameter | Description | Required/ Optional |
---|---|---|
mId | Merchant identifier. | Required |
orderId | Order identifier. | Required |
Response parameters
Parameter | Type | Description |
---|---|---|
id | string | Merchant identifier. |
orderRefid | string | Reference identifier of the order fee with which the service charge is associated |
name | string | Name of the order fee. |
price | integer | Order fee price. |
note | string | Comments or information, if any. |
createdTime | integer | Time when the service charge was recorded on the server. |
orderClientCreatedTime | integer | Order client-created time for user data of line item. |
percentage | integer | Service charge amount in percent. |
orderFeeid | string | Order fee identifier. |
Remove service charges associated with a merchant from an order
To remove one or more service charges associated with a merchant from an order:
- Send a DELETE request to
/v3/merchants/{mId}/orders/{orderId}/order_fee_line_items/{orderFeeLineItemId}
. - Enter the merchant identifier (
mId
), order identifier (orderId
) and the order fee line item identifier (orderFeeLineitemId
) in the request.
curl --request DELETE \
--url 'https://apisandbox.dev.clover.com/v3/merchants/{mId}/orders/{orderId}/order_fee_line_items/{orderFeeLineItemId}' \
--header 'content-type: application/json' \
--header 'Authorization: Bearer {access_token}'
Request parameters
Parameter | Type | Description | Required/ Optional |
---|---|---|---|
mId | string | Merchant identifier. | Required |
orderId | string | Order identifier. | Required |
orderFeeLineItemId | string | Order fee line item identifier. | Required |
Response parameters
Message | Type | Description |
---|---|---|
200 | string | Successful response. Service charge is deleted. |
Updated 7 months ago