Build apps for Clover Station Duo 2: Dual touch screen device
Merchants who want to increase sales completion efficiency with a dedicated customer-facing display can use the Station Duo 2. Station Duo 2 splits the display unit of a single device into two terminals - customer-facing and merchant-facing displays, thus reducing hardware redundancy.
Station Duo 2 components
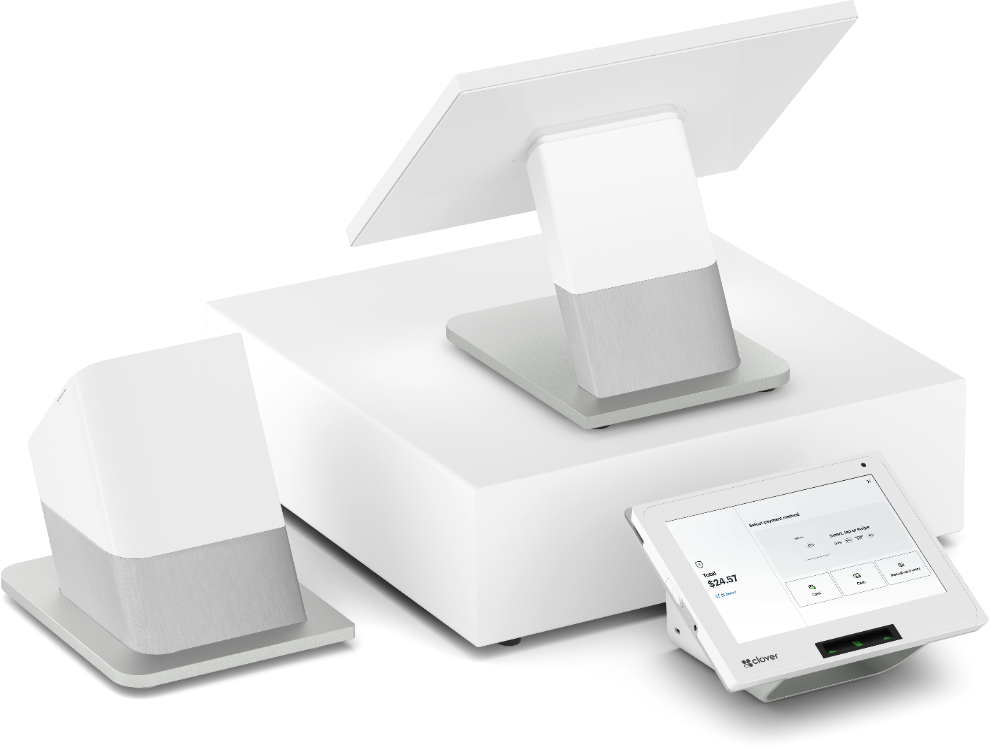
Station Duo 2 comprises the following components:
-
Customer-facing screen—An 8-inch HD display that is similar to the Clover Mini 3 and includes a relocated card slot for faster, more intuitive payments.
-
Merchant-facing screen—A 14-inch FHD display that tilts to allow viewing from a range of heights. The merchant-facing display tilts but does not swivel.
-
Printer—Standalone receipt printer.
-
Special cover glass—Both displays have chemically strengthened cover glass with anti-fingerprint coating.
Build apps
Apps launch on the merchant-facing display. If your app runs on Clover Station 2018 or Station Duo 1, the app should run on Station Duo 2 when you adjust for the following:
- Android API Level:
- Station 2018: 24
- Station Duo 1: 27
- Station Duo 2: 29
- Payment interfaces: The merchant-facing display does not provide EMV chip, NFC, or MSR technologies. Initiate payments with the Clover API. The payment screen appears on the customer-facing terminal and supports EMV chip, NFC, and MSR technologies.
- Platform class: Use the
Platform2
class or standard Android APIs to detect system features and capabilities.
Apps that run on the customer-facing display, Station 2018, or Station Duo 1 may need changes to function properly on the Duo 2. Purchase a Dev Kit to test your apps.
Build and launch apps on the customer-facing display using:
- Custom tenders
- Customer-facing platform—Use the Pay Display framework to build and launch apps on the customer-facing display.
Work with Station Duo 2 hardware
1. Use payment interfaces
The customer-facing display includes an EMV chip card reader, NFC reader, and MSR reader. Customers can directly swipe gift and loyalty cards and complete the purchase.
2. Use the cameras
Station Duo 2 includes two cameras:
Camera | ID |
---|---|
Customer-facing camera (default camera) | 0 |
Merchant-facing camera | 1 |
To capture an image with a camera, use the Android MediaStore.ACTION_IMAGE_CAPTURE
intent.
static int CAMERA_ID_MERCHANT_FACING = 1;
static int CAMERA_ID_CUSTOMER_FACING = 0;
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
intent.putExtra("android.intent.extra.CAMERA_ID", CAMERA_ID_MERCHANT_FACING);
startActivity(cameraIntent);
3. Scan barcodes
You can use both the customer-facing and merchant-facing displays to scan barcodes. The camera on the merchant-facing display is the default barcode scanner. The device displays a scanner preview window while scanning a barcode.
BarcodeScanner barcodeScanner = new BarcodeScanner(context);
List<Integer> available = barcodeScanner.getAvailable();
Bundle extras = new Bundle();
if (available.contains(BarcodeScanner.BARCODE_SCANNER_FACING_CUSTOMER)) {
extras.putInt(Intents.EXTRA_SCANNER_FACING, BarcodeScanner.BARCODE_SCANNER_FACING_CUSTOMER);
}
barcodeScanner.startScan(extras);
4. Use a printer
The Station Duo 2 includes an external USB-connected printer without a display screen.
PrinterConnector.getPrinters(Category)
PrinterConnector.getPrinterTypeDetails(Printer)
5. Use the speakers
Both the customer-facing and merchant-facing displays have speakers. By default, the speakers on the merchant-facing display play the audio.
To play audio on the customer-facing display, set the Android AudioAttributes.USAGE_VOICE_COMMUNICATION_SIGNALLING
value.
MediaPlayer mediaPlayer = new MediaPlayer();
AudioAttributes attrs = new AudioAttributes.Builder().setUsage(
AudioAttributes.USAGE_VOICE_COMMUNICATION_SIGNALLING).build();
mediaPlayer.setAudioAttributes(attrs);
NOTE
For any user-interface actions, such as tap a button, audio is played from the device where the action is completed.
6. Use the microphones
Both the customer-facing and merchant-facing displays have microphones. By default, the merchant-facing display is used for all audio input. This functionality is set in the Android AudioSource.MIC
value.
To use the microphone on the customer-facing terminal, set the AudioSource.CAMCORDER
value.
MediaRecorder recorder = new MediaRecorder();
recorder.setAudioSouce(MediaRecorder.AudioSource.CAMCORDER);
Build with the Platform2 class
The Platform2
class replaced the Platform class in the Clover Android SDK. If you have used the com.clover.sdk.util.Platform
class, then you need to rebuild your app with the latest Clover Android SDK to get the com.clover.sdk.util.Platform2
class functionality to develop apps on Station Duo 2.
The Platform2
class in the Clover Android SDK lets you discover Clover-specific device features and capabilities. We recommend that you:
- Use Platform2 or the standard Android functions to discover device features.
- Do not hardcode application behavior based on correlated characteristics such as Android API level or model name.
For example, if a Clover device supports the system feature FEATURE_TELEPHONY
, it supports mobile data.
boolean mobileData = context.getPackageManager()
.hasSystemFeature(PackageManager.FEATURE_TELEPHONY);
Build customer-facing apps
You can build customer-facing apps on Station Duo 2 only with custom tenders or the customer-facing platform. The configuration required to make sure that your customer-facing apps run on any configuration are as follows:
1. Station Duo 2 activity lifecycle
Android activities on the merchant-facing display work normally as described by the Android activity documentation.
Activities on the customer-facing display follow a slightly modified lifecycle; immediately after onResume
is invoked, the onPause
method is invoked, the app remains in this paused state while being used by the customer until it is no longer visible. You may need to move all code out of onResume
and onPause
and into onStart
and onStop
instead. This change should generally be safe for all Clover devices.
If you want a different behavior for Activities running on the customer-facing display of Station Duo 2 versus running on the primary display of devices like Clover Mini, use the method CustomerMode.isShownOnPrimaryDisplay(Activity)
to detect if your Activity is running on a secondary-display that exhibits the alternate lifecycle behavior.
TIP
On the Station Duo 2 customer-facing display, an Android Activity window and components in it cannot have focus. The Activity, however, is still usable by the customer.
2. Customer mode
The CustomerMode
class allows an app to hide the Android status bar and the navigation bar for the purpose of developing customer-facing experiences. This class has been available since the release of Clover Mini and Mobile. By default, these bars are not present on Station Duo’s customer-facing display.
If you are using customer mode for customer-facing apps deployed to older Clover devices and you intend those apps to work on Station Duo 2, you may need to rebuild your app with a more recent version of the Clover Android SDK, as there were changes made to the CustomerMode
class in mid-2019.
TIP
The Station Duo 2 merchant-facing display does not swivel. If you are using customer mode on the merchant-facing display you should consider whether this functionality is desired on Station Duo 2. If so, ensure that merchants can exit customer mode so the merchant operator does not end up trapped in your app.
3. Keyboard or Input Method Editor (IME)
Since activities on the customer-facing display cannot have focus, you cannot use Android IME keyboard to accept input. Instead, if your customer-facing app requires a soft keyboard, use the Clover Android SDK provided Intents.ACTION_KEYPAD
Activity to gather input from the user.
4. Small pop-up or Toast messages
Toast messages provides simple feedback about an operation in a small pop-up. These messages can only display on apps on the merchant-facing display. You cannot use toast messages on the customer-facing display. For apps running on the customer-facing display, use other user interface components to display messages.
Updated 10 days ago