Pre-auth operations
Pre-auth operations include the following:
Accept a pre-auth
Use the PreAuthRequestIntentBuilder
class to build an Intent to start an activity that will guide a user through a pre-auth payment steps. The resulting pre-auth payment needs to be captured with a CapturePreAuth Request. The steps may include payment confirmation and receipt selection. These options can be customized through the merchant settings or by using options that can be configured on the PreAuthRequestIntentBuilder
.
Build a pre-auth request
You can build a pre-auth request with a few lines of code.
val paymentId = "<posPaymentId>" // should be unique for each request
val amount = 5000L
val context = this
val builder = PreAuthRequestIntentBuilder(paymentId, amount)
val intent = builder.build(context)
Context context = this;
String externalPaymentId = "<posPaymentId>"; // should be unique for each request
Long amount = 5000L;
PreAuthRequestIntentBuilder builder = new PreAuthRequestIntentBuilder(externalPaymentId, amount);
Intent intent = builder.build(context);
The Intent can then be used to start the activity to process the pre-auth request.
A few options can be set on the builder to provide additional processing instructions:
- CardOptions—settings for supported card entry methods and card confirmations
- TokenizeOptions—settings for pay+tokenize card for use in e-commerce services
CardOptions
The CardOptions
class provides a static method to optionally set the supported card entry methods, options to auto-accept duplicate payment challenges, and a cardNotPresent flag.
Static Method | Description |
---|---|
Instance( cardEntryMethods : Set<CardEntryMethod>, cardNotPresent : Boolean, autoAcceptDuplicates : Boolean) | Optionally set any of these flags to create a CardOptions instance. If null is passed, the default settings for the merchant will be used. |
Example—Set the card entry method to Manual only and auto-accept duplicate challenges
val builder = PreAuthRequestIntentBuilder("<posPaymentId>", 5000)
builder.cardOptions(
PreAuthRequestIntentBuilder.CardOptions.Instance(
CardEntryMethod.Manual(), // manual only
null, // card not present
true)) // auto-accept duplicates
val intent = builder.build(context)
PreAuthRequestIntentBuilder builder = new PreAuthRequestIntentBuilder("<posPaymentId>", 5000);
builder.cardOptions(
PreAuthRequestIntentBuilder.CardOptions.Instance(
CardEntryMethod.Manual(), // manual only
null, // card not present
true)); // auto-accept duplicates
Intent intent = builder.build(context);
TokenizeOptions
The TokenizeOptions
class contains a single property, suppressConfirmation. However, the presence of TokenizeOptions, regardless of the suppressConfirmation value, indicates that a token for the payment card used should be generated. The tokenize option is secondary to the payment, so, a failed tokenization could still result in a successful payment but a failed payment will not result in a successful tokenization.
Value | Description |
---|---|
Instance(suppressConfirmation:boolean) | Use to create a TokenizeOptions instance where confirmation may be required. By default, confirmation is required and is requested. |
NOTE
Your device must have core-payments installed. If your device does not have core-payments installed, please contact support if you would like to utilize this feature.
Example—Request a payment token with a payment
val builder = PreAuthRequestIntentBuilder("<posPaymentId>", 5000)
builder.tokenizeOptions(PreAuthRequestIntentBuilder.TokenizeOptions.Instance(
false // suppressConfirmation
));
val intent = builder.build(context)
PreAuthRequestIntentBuilder builder = new PreAuthRequestIntentBuilder("<posPaymentId>", 5000);
builder.tokenizeOptions(PreAuthRequestIntentBuilder.TokenizeOptions.Instance(
false // suppressConfirmation
));
Intent intent = builder.build(context);
Additional Fields
You can set additional fields on the PreAuthRequestIntentBuilder
:
Field | Description |
---|---|
externalPaymentId: String | Custom external payment identifier (Id) that persisted with the transaction. |
amount: Long | Total amount paid. |
externalReferenceId: String | Unique identifier (Id), such as an invoice or purchase order (PO) number, that is sent to the merchant's gateway and displayed in settlement records. Format: Supported for US—alphanumeric characters with in-between spaces Length: Maximum 12, including spaces and alphanumeric characters |
Examples—Custom card entry methods
These examples set the card entry methods to Manual, Swipe, and NFC only.
val builder = PreAuthRequestIntentBuilder("<posPaymentId>", 5000)
var cardEntryMethods = setOf<CardEntryMethod>(
CardEntryMethod.MANUAL,
CardEntryMethod.MAG_STRIPE,
CardEntryMethod.NFC)
var cardOptions = PreAuthRequestIntentBuilder.CardOptions.Instance(
cardEntryMethods,
null, // card not present
null) // auto accept duplicates
builder.cardOptions(cardOptions)
val intent = builder.build(context)
PreAuthRequestIntentBuilder builder = new PreAuthRequestIntentBuilder("<posPaymentId>", 5000);
Set<CardEntryMethod> cardEntryMethods = new HashSet<>();
cardEntryMethods.add(CardEntryMethod.MANUAL);
cardEntryMethods.add(CardEntryMethod.NFC);
PreAuthRequestIntentBuilder.CardOptions cardOptions = PreAuthRequestIntentBuilder.CardOptions.Instance(
cardEntryMethods,
null, //card not present
null); //auto accept duplicates
builder.cardOptions(cardOptions);
Intent intent = builder.build(context);
Capture a pre-auth
Use the CapturePreAuthRequestIntentBuilder
class to build an Intent to start an activity that will guide a user through the capturing a pre-auth steps. The steps may include tip selection, signature collection, payment confirmation, and receipt selection. These options can be customized through the merchant settings or by using options that can be configured on the CapturePreAuthRequestIntentBuilder
.
Build a capture pre-auth request
You can build a pre-auth request with a few lines of code.
val paymentId = payment.id // id of a preauth payment
val amount = 1200L // capture amount
val context = this
val builder = CapturePreAuthRequestIntentBuilder(paymentId, amount)
val intent = builder.build(context)
Context context = this;
String paymentId = payment.id; // id of a preauth payment
Long amount = 1200L; // capture amount
CapturePreAuthRequestIntentBuilder builder = new CapturePreAuthRequestIntentBuilder(paymentId, amount);
Intent intent = builder.build(context);
The Intent can then be used to start the activity to process the capture pre-auth request.
There are several options that can be set on the builder to provide additional processing instructions:
- TipOptions & SignatureOptions—settings for tips (if tips are enabled for merchant) and settings for signature requirements
- ReceiptOptions—settings for receipt screen
TipOptions and SignatureOptions
TipOptions and SignatureOptions are independent options that can be excluded, however, they share the same location
value (on screen/on paper).
TipOptions
The TipOptions class provides static helper methods to simplify the creation of TipOptions.
Method | Description |
---|---|
Disable() | Set the options so no tip is requested. |
Provided(tipAmount: Long) | If the tip amount is known before the payment request, this adda the tip amount to the payment and the payment is finalized. |
PromptCustomer( baseAmount: Long, tipSuggestions: List<TipSuggestion>) | Use to set the amount used to calculate the percentage-based tips or override the tip suggestions with fixed amounts or custom percentages. |
NOTE
The merchant’s payment gateway settings must be configured for tippable payment for a tip-adjustable payment to be created. On some devices, tips are taken prior to the payment and can be supported without merchant payment gateway settings, as the payment will be finalized by the gateway.
SignatureOptions
The SignatureOptions
class provides static helper methods to simplify the creation of SignatureOptions.
Method | Description |
---|---|
Disable() | Set the options so no signature is requested. |
PromptCustomer() | Use to override the merchant settings for when and if, to prompt for a signature. |
Example—Disable tip and signature for a payment
val builder = CapturePreAuthRequestIntentBuilder("<posPaymentId>", 1200)
builder.tipAndSignatureOptions(
CapturePreAuthRequestIntentBuilder.TipOptions.Disable(),
CapturePreAuthRequestIntentBuilder.SignatureOptions.Disable(),
null) // preferOnScreen
val intent = builder.build(context)
CapturePreAuthRequestIntentBuilder builder = new CapturePreAuthRequestIntentBuilder("<posPaymentId>", 1200);
builder.tipAndSignatureOptions(
CapturePreAuthRequestIntentBuilder.TipOptions.Disable(),
CapturePreAuthRequestIntentBuilder.SignatureOptions.Disable(),
null); // preferOnScreen
Intent intent = builder.build(context);
ReceiptOptions
The ReceiptOptions
class provides the following options:
Static Method | Description |
---|---|
Default(cloverShouldHandleReceipts: Boolean) | Set whether Clover should handle printing or sending SMS/Email receipts. |
skipReceiptSelection:Boolean() | Configure the flow to skip the receipt selection. |
Instance( cloverShouldHandleReceipts : Boolean, smsReceiptOption : SmsReceiptOption, emailReceiptOption : EmailReceiptOption, printReceiptOption : PrintReceiptOption, noReceiptOption : NoReceiptOption) | Disable or enable every receipt selection button on the receipt screen. In addition, this can be used to decide if Clover should handle the printing or sending of SMS/Email receipts. |
Example—Disable the SMS and email receipt buttons and set cloverShouldHandleReceipts
to true.
cloverShouldHandleReceipts
to true.val amount = 1000L
val paymentId = "<paymentId-of-preauth-to-be-captured>"
val builder = CapturePreAuthRequestIntentBuilder(paymentId, amount)
val receiptOptions = CapturePreAuthRequestIntentBuilder.ReceiptOptions.Instance(
true,
CapturePreAuthRequestIntentBuilder.ReceiptOptions.SmsReceiptOption.Disable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.EmailReceiptOption.Disable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.PrintReceiptOption.Enable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.NoReceiptOption.Enable())
val capturePreauthRequestIntent = builder.receiptOptions(receiptOptions).build(context)
Long amount = 1000L;
String paymentId = "<paymentId-of-preauth-to-be-captured>";
CapturePreAuthRequestIntentBuilder builder = new CapturePreAuthRequestIntentBuilder(paymentId, amount);
CapturePreAuthRequestIntentBuilder.ReceiptOptions receiptOptions = CapturePreAuthRequestIntentBuilder.ReceiptOptions.Instance(
true,
CapturePreAuthRequestIntentBuilder.ReceiptOptions.SmsReceiptOption.Disable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.EmailReceiptOption.Disable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.PrintReceiptOption.Enable(),
CapturePreAuthRequestIntentBuilder.ReceiptOptions.NoReceiptOption.Enable());
Intent capturePreauthRequestIntent = builder.receiptOptions(receiptOptions).build(context);
Example on device—No SMS or email receipt
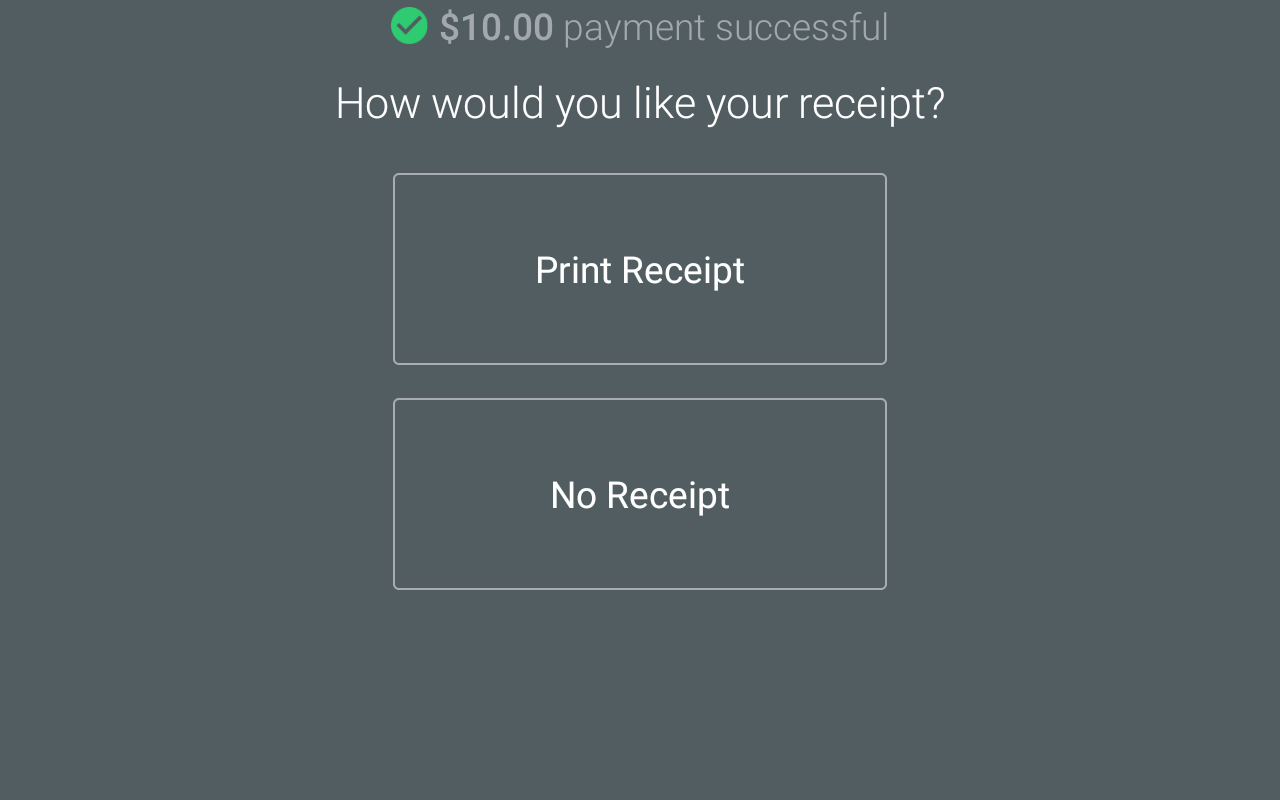
Additional fields
Field | Description |
---|---|
amount: Long | Amount to capture. |
paymentId: String | Payment identifier (Id) of the pre-auth to capture. |
Examples
Example custom tips—Create custom tip options of $1.00, $2.00, and 20% and prefer onScreen tips.
val builder = CapturePreAuthRequestIntentBuilder("<posPaymentId>", 1200)
var tipSuggestions = listOf<TipSuggestion>(
TipSuggestion.Amount("Thanks!", 100),
TipSuggestion.Amount("Good Job!", 200),
TipSuggestion.Percentage("Great!", 20)
)
var tipOptions = CapturePreAuthRequestIntentBuilder.TipOptions.PromptCustomer(null, tipSuggestions)
builder.tipAndSignatureOptions(
tipOptions,
null, // signature options
true) // preferOnScreen
val intent = builder.build(context)
Example minimal interaction—Reduce the screens to only the payment screen. This example disables tips, signatures, and payment confirmations, and skips the receipt screen.
val builder = CapturePreAuthRequestIntentBuilder("<paymentId>", 1200)
builder.tipAndSignatureOptions(
CapturePreAuthRequestIntentBuilder.TipOptions.Disable(),
CapturePreAuthRequestIntentBuilder.SignatureOptions.Disable(),
true)
builder.receiptOptions(CapturePreAuthRequestIntentBuilder.ReceiptOptions.SkipReceiptSelection())
CapturePreAuthRequestIntentBuilder builder = new CapturePreAuthRequestIntentBuilder("<paymentId-of-preauth-to-be-captured>", 1200);
builder.tipAndSignatureOptions(
CapturePreAuthRequestIntentBuilder.TipOptions.Disable(),
CapturePreAuthRequestIntentBuilder.SignatureOptions.Disable(),
true);
builder.receiptOptions(CapturePreAuthRequestIntentBuilder.ReceiptOptions.SkipReceiptSelection());
Intent intent = builder.build(context);
Set incremental auths
Use the IncrementalAuthRequestIntentBuilder
class to build an Intent to start an activity to increase the authorized amount of a transaction to verify the cardholder has sufficient funds for the purchase.
NOTE
Incremental authorizations are only available for:
DiscoverⓇ, MastercardⓇ, and VisaⓇ cards, and Merchant types—aircraft rentals, amusement parks, bicycle rentals, boat rentals, car rentals, cruise lines, drinking places, eating places, equipment rentals, lodgings, motor home rentals, motorcycle rentals, trailer parks/campgrounds, and truck rentals.
You can build an incremental auth request with a few lines of code.
Example—Incremental auth request
The following example shows how to build an incremental auth request.
val paymentId = payment.id // id of a preauth payment
val amount = 1200L // increment amount
val context = this
val builder = IncrementalAuthRequestIntentBuilder(paymentId, amount)
val intent = builder.build(context)
String paymentId = payment.getId(); // id of a preauth payment
Long amount = 1200L; // increment amount
Context context = this;
IncrementalAuthRequestIntentBuilder builder = new IncrementalAuthRequestIntentBuilder(paymentId, amount);
Intent intent = builder.build(context);
Request fields
You can set additional fields on IncrementalAuthRequestIntentBuilder
:
Field | Description |
---|---|
amount: Long | Amount to increment. |
paymentId: String | Payment identifier (Id) of the pre-auth to increment. |
Response details
- Upon completion of the incremental auth, the response comes through
onActivityResult()
. - When the incremental auth request is successful, you can retrieve the Payment object with
Intents.EXTRA_PAYMENT
. The new Payment object should reflect the incremented amount. - When the request is unsuccessful, you can retrieve the failure message with
Intents.EXTRA_FAILURE_MESSAGE
.
Example—Incremental auth response
fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == INCREMENTAL_AUTH_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
val payment: Payment = data.getParcelableExtra(Intents.EXTRA_PAYMENT)
} else {
// incremental auth failed, check for error
val failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE)
}
}
}
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == INCREMENTAL_AUTH_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
Payment payment = data.getParcelableExtra(Intents.EXTRA_PAYMENT);
} else {
// incremental auth failed, check for error
String failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE);
}
}
}
Updated 10 months ago