Per-transaction settings
With version 1.2.0 and above of the software development kit (SDK), you can override certain merchant-level settings for specific transactions. These per-transaction settings give you greater control over tips, signatures, receipts, duplicate transaction logic, offline payments, payment confirmations, and other functions.
Regional rules and inherited settings
For each transaction, several checks are applied by the device to ensure the correct customer-facing screens display. Generally, transaction settings override any device-specific or merchant-configured settings. An exception is if the merchant is operating in a region where certain rules cannot be overridden due to legal or regulatory requirements.
For example, regional rules override some SignatureEntryLocation
options. The following is a sample of a sale transaction that is set to not require a signature:
private SaleRequest setupSaleRequest() {
SaleRequest saleRequest = new SaleRequest();
saleRequest.setExternalId(ExternalIdUtils.generateNewID());
saleRequest.setAmount(2000L);
saleRequest.setSignatureEntryLocation(DataEntryLocation.NONE);
return saleRequest;
}
- If this request is processed by a US merchant, the signature screen does not appear in the payment flow.
- If the request is processed in Argentina, a regional rule requiring signatures is applied, and the signature screen appears despite the transaction setting.
The following image illustrates how various checks are made against transaction settings. In this flow, the regional rules checked in the final step override any other settings that may otherwise apply to the transaction.
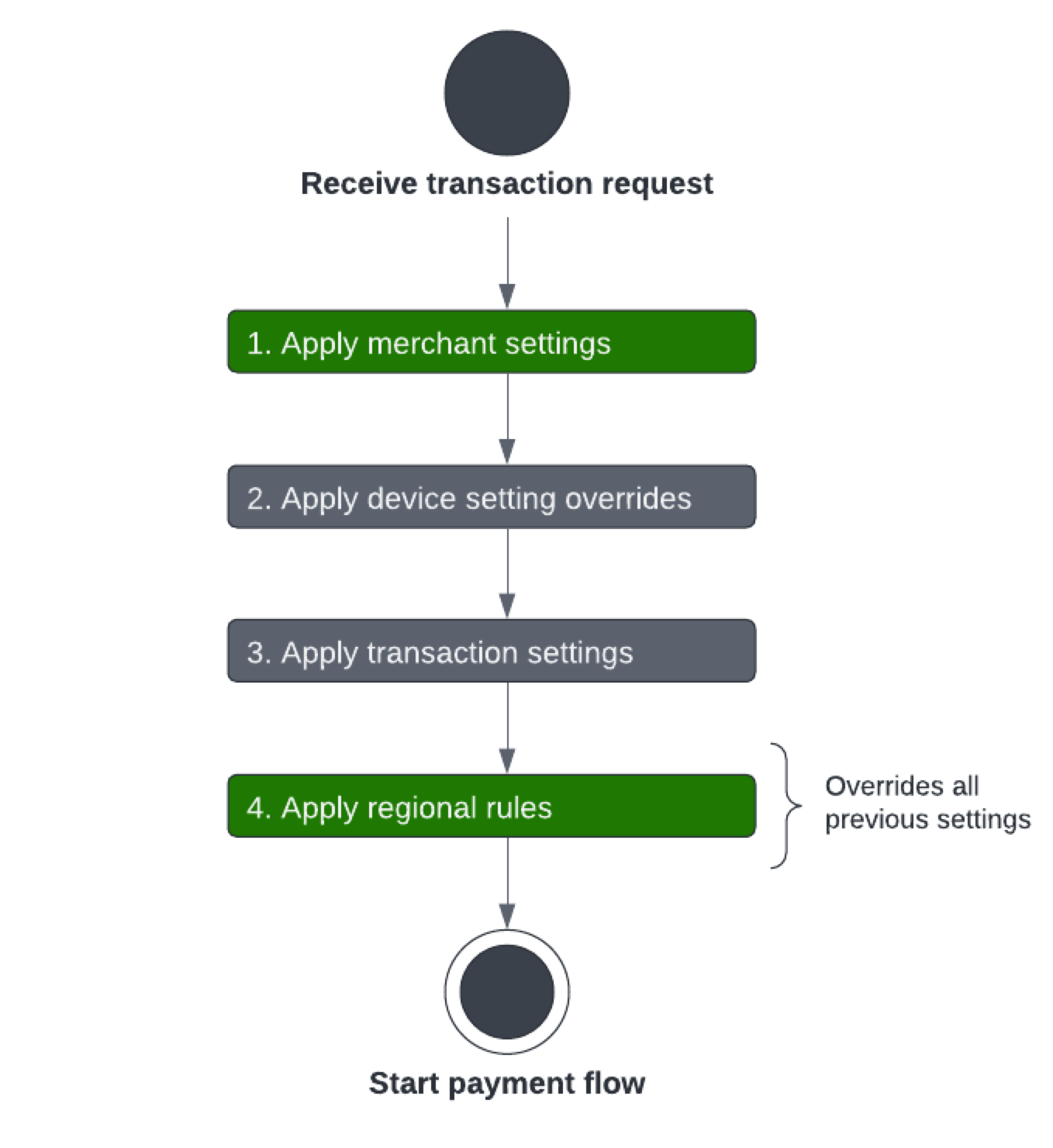
Image to illustrate the checks made against transaction settings
Important
To use transaction settings, you must initiate a subclass of
TransactionRequest
(that is, aSaleRequest
,AuthRequest
, orPreAuthRequest
). Transaction settings work for all subclasses ofTransactionRequest
, but each may only allow the modification of some settings. Support for each setting may also be different for each SDK.
Tips
TipMode (TipMode enum)
The tipMode
setting indicates the location from which to accept the tip. You can also omit the tip.
The values include:
Value | Description | Request Methods | Devices |
TIP_PROVIDED |
Tip amount is included in the request
You must also set the TipAmount field on the request. |
SaleRequest |
Clover Flex, Clover Mini, Clover Mobile, and Clover Station |
ON_SCREEN_BEFORE_PAYMENT |
Tip screen displays on the Clover device before payment. | SaleRequest |
Clover Flex, Clover Mini, and Clover Mobile |
ON_SCREEN_AFTER_PAYMENT |
Tip screen displays on the Clover device after payment. | AuthRequest |
Clover Station or Clover Station 2018 |
NO_TIP |
Tip is not requested for the payment, that is the tip screen is skipped during the payment flow. This setting overrides the merchant setting for tips. | SaleRequest |
Clover Flex, Clover Mini, Clover Mobile, and Clover Station |
TippableAmount (Long)
This option sets the amount to use when calculating tips, for example, saleRequest.tippableAmount = 2000L;
. This setting is useful if you want to present customers with the option to tip for a portion of the total. When the ADD_TIP
screen appears during payment, this amount displays in the Tip based on
field.
TipSuggestions (Array)
This setting allows you to override the default amounts and labels for tip suggestions that display on the ADD_TIP
screen. Each suggestion can set three values:
- a
percentage
oramount
- an
isEnabled
indicator - a
name
label
You can include a maximum of four suggestions. The following examples illustrate a few suggestions and the screens that appear.
1. Four custom tip percentage suggestions
const tipSuggestion1 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion1.setPercentage(18);
tipSuggestion1.setIsEnabled(true);
tipSuggestion1.setName("Acceptable");
const tipSuggestion2 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion2.setPercentage(20);
tipSuggestion2.setIsEnabled(true);
tipSuggestion2.setName("Good");
const tipSuggestion3 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion3.setPercentage(25);
tipSuggestion3.setIsEnabled(true);
tipSuggestion3.setName("Great");
const tipSuggestion4 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion4.setPercentage(30);
tipSuggestion4.setIsEnabled(true);
tipSuggestion4.setName("Excellent");
saleRequest.setTipSuggestions([tipSuggestion1, tipSuggestion2, tipSuggestion3, tipSuggestion4]);
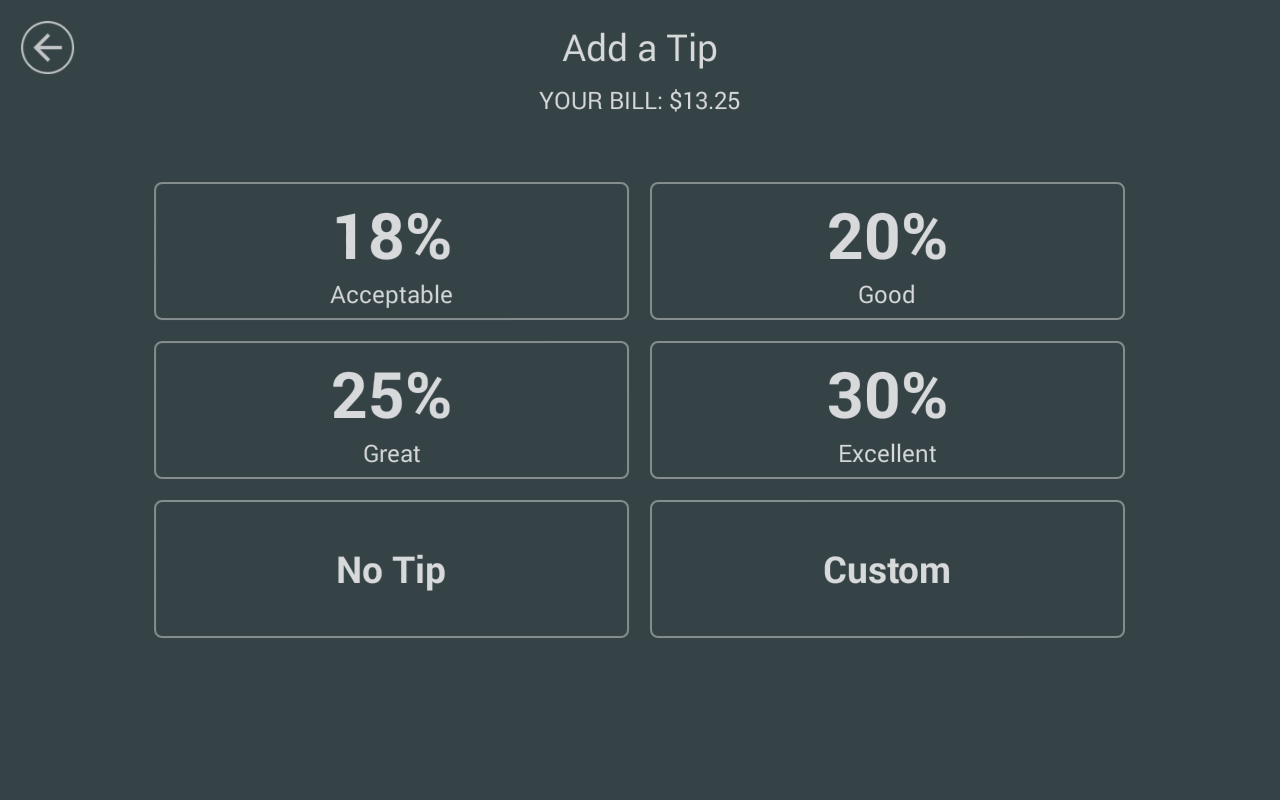
Four custom tip percentage suggestions
2. Two custom tip percentage suggestions
const tipSuggestion1 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion1.setPercentage(15);
tipSuggestion1.setIsEnabled(true);
tipSuggestion1.setName("Good");
const tipSuggestion2 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion2.setPercentage(20);
tipSuggestion2.setIsEnabled(true);
tipSuggestion2.setName("Great");
saleRequest.setTipSuggestions([tipSuggestion1, tipSuggestion2, null, null]);
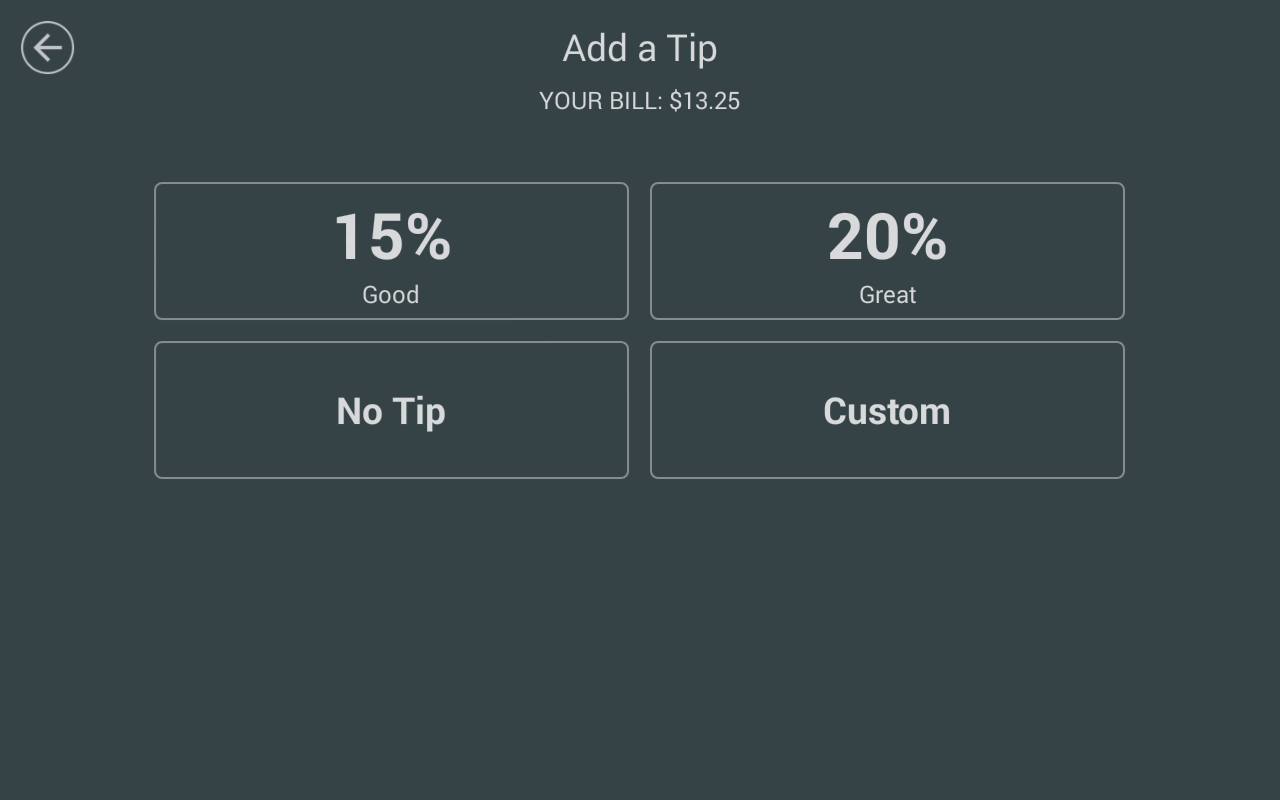
Two custom tip percentage suggestions
3. Four custom tip amount suggestions
If the merchant wants tip options in whole dollar amounts, you can set an amount instead of a percentage. You cannot disable or override the No Tip and Custom buttons with this setting.
const tipSuggestion1 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion1.setAmount(100);
tipSuggestion1.setIsEnabled(true);
tipSuggestion1.setName("Acceptable 😌");
const tipSuggestion2 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion2.setAmount(200);
tipSuggestion2.setIsEnabled(true);
tipSuggestion2.setName("Good 😊");
const tipSuggestion3 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion3.setAmount(300);
tipSuggestion3.setIsEnabled(true);
tipSuggestion3.setName("Great 😛");
const tipSuggestion4 = new clover.sdk.merchant.TipSuggestion();
tipSuggestion4.setAmount(400);
tipSuggestion4.setIsEnabled(true);
tipSuggestion4.setName("Excellent 😍");
saleRequest.setTipSuggestions([tipSuggestion1, tipSuggestion2, tipSuggestion3, tipSuggestion4]);
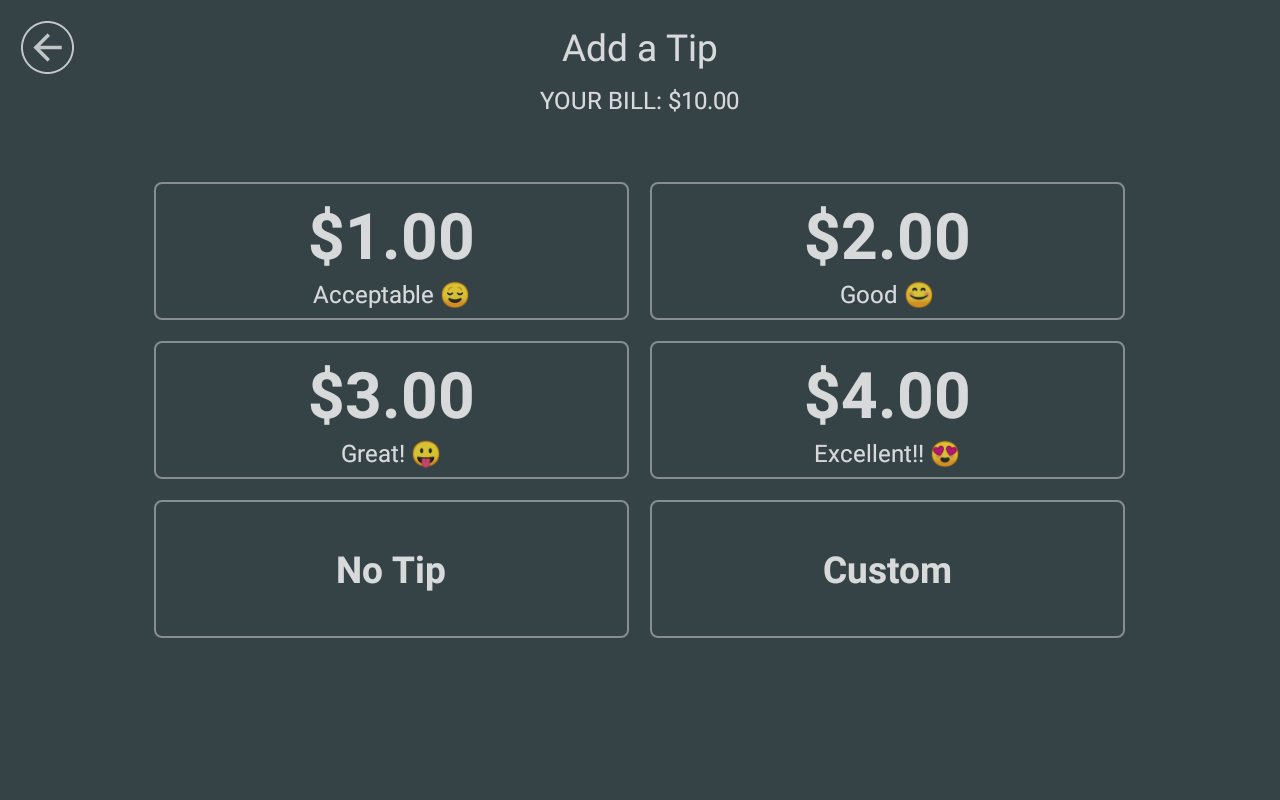
Four custom tip amount suggestions
Signatures
SignatureThreshold (Long)
This setting allows you to override the merchant-configured signature threshold, which is the minimum amount for requiring a customer signature.
For example:
- To require a signature for any transaction greater than $25.00, set the
SignatureThreshold
to2500
:
saleRequest.signatureThreshold = 2500;
- To require a signature for any amount, set the
SignatureThreshold
to0
:
saleRequest.signatureThreshold(0);
saleRequest.signatureThreshold(0) - To override the signature threshold entirely so that a signature is never required, set
SignatureEntryLocation
toNONE
.
SignatureEntryLocation (DataEntryLocation enum)
This setting allows you to override the merchant-level Signature Entry Location. For example, to force the Clover device to take the signature on-screen, use: saleRequest.signatureEntryLocation = DataEntryLocation.ON_SCREEN;
The values are:
Value | Description |
---|---|
ON_SCREEN | Takes the signature on the Clover device screen. |
ON_PAPER | Takes the signature on the paper receipt. |
NONE | Skips the signature request for the transaction. |
AutoAcceptSignature (Boolean)
This setting allows you to automatically accept a signature—on screen or on paper—for the given transaction, if applicable. The override prevents the SDK from transmitting signature confirmation requests back to the calling program. The transaction continues processing as if the caller initiated an acceptSignature()
request:
saleRequest.autoAcceptSignature = true;
Receipts
DisableReceiptSelection (Boolean)
This setting bypasses the customer-facing receipt selection screen. For example, use this setting if you plan to print receipts from a non-Clover printer: saleRequest.disableReceiptSelection = true;
Duplicate checking
DisableDuplicateChecking (Boolean)
The saleRequest.disableDuplicateChecking = true;
setting bypasses logic that checks for potential duplicate transactions—payments made with the same card type and last 4 digits within the same hour—and asks the merchant to confirm acceptance of the payments. Disabling duplicate checking makes your transactions faster but increases the risk associated with a potential duplicate payment.
Offline payments
The Clover device records offline payments when a merchant attempts a transaction without a network or Wi-Fi connection. In this scenario, the device also can't verify the payment gateway or check if the card is valid and has sufficient funds.
By default, Clover Station, Station 2018, Mini, Mobile, and Flex are set to take offline payments for up to 7 days. Merchants can disable offline payments if they don't want to accept the associated risk, or they can set limits for offline payments based on the transaction amount or the total amount processed in an offline state. See the merchant help page for more information.
Override merchant settings for offline transactions
The Remote Pay SDK provides three settings that can override the merchant’s settings for offline payments. The settings are based on the level of risk the merchant must accept if an offline payment is eventually declined.
Important
Allowing any offline payment with one of the following per-transaction settings overrides any merchant-configured limits on these riskier transactions. This includes the limit on days before going online, as well as the amount limit on each transaction and the total offline payments limit.
By using any of the offline payments settings, you assume responsibility for warning the merchant of any possible risk and enforcing any limits on offline transactions required for the merchant.
AllowOfflinePayment (Boolean)—Least risk
If the merchant disables offline payments, setting AllowOfflinePayment = true;
overrides the merchant's settings. If the device is offline and a payment is attempted, the merchant is prompted to accept or decline the payment with an offline challenge.
To set the saleRequest
to allow an offline payment if the device cannot connect to the payment gateway: saleRequest.allowOfflinePayment = true;
ApproveOfflinePaymentWithoutPrompt (Boolean)—More risk
When saleRequest.approveOfflinePaymentWithoutPrompt = true;
, offline payments are approved without a challenge. In this case, the merchant may not know that a payment was taken offline. Transactions complete faster with this setting. However, implement this setting only if you are sure the merchants using your integration are willing to accept the risk associated with offline payments.
Note
You can also confirm offline payments automatically with the
AutoAcceptPaymentConfirmations
per-transaction setting.
ForceOfflinePayment (Boolean)—Most risk
When saleRequest.forceOfflinePayment = true;
the device takes payments offline regardless of connection status, and overrides any offline payment limits set by the merchant. The device processes transactions faster because it doesn't prompt the merchant to accept or reject the payment. This setting is useful for merchants that need to complete transactions as quickly as possible. However, implement this setting only if you are sure the merchants using your integration are willing to accept the risk associated with offline payments.
Payment confirmation settings
AutoAcceptPaymentConfirmations (Boolean)
The saleRequest.autoAcceptPaymentConfirmations = true;
allows you to automatically accept any payment confirmation requests triggered by challenges encountered at the payment gateway, for example, an offline payment or potential duplicate payment. The override prevents the SDK from transmitting payment confirmation requests back to the calling program. The transaction continues processing as if the caller initiated a confirmPayment()
request.
Automatically accepting payment confirmation requests makes your transactions faster. However, implement this setting only if you are sure the merchants using your integration are willing to accept the risk associated with offline payments and potential duplicate payments.
Other functions
DisableCashback (Boolean)
This setting disables cashback functionality:
saleRequest.disableCashback = true;
DisablePrinting (Boolean)
This setting disables automatic printing of receipts for transactions:
saleRequest.disablePrinting = true;
DisableRestartTransactionOnFail (Boolean)
This setting prevents the Clover device from restarting a transaction that times out or is declined:
saleRequest.disableRestartTransactionOnFail = true;
CardEntryMethods
This setting determines the card entry options that display for the customer on the start screen.
Card entry values include:
CARD_ENTRY_METHOD_MAG_STRIPE
CARD_ENTRY_METHOD_ICC_CONTACT
CARD_ENTRY_METHOD_NFC_CONTACTLESS
CARD_ENTRY_METHOD_MANUAL
- If no card entry options are set, all payment methods display by default.
The following image illustrates each CardEntryMethod
option on the Clover Mini START
screen.
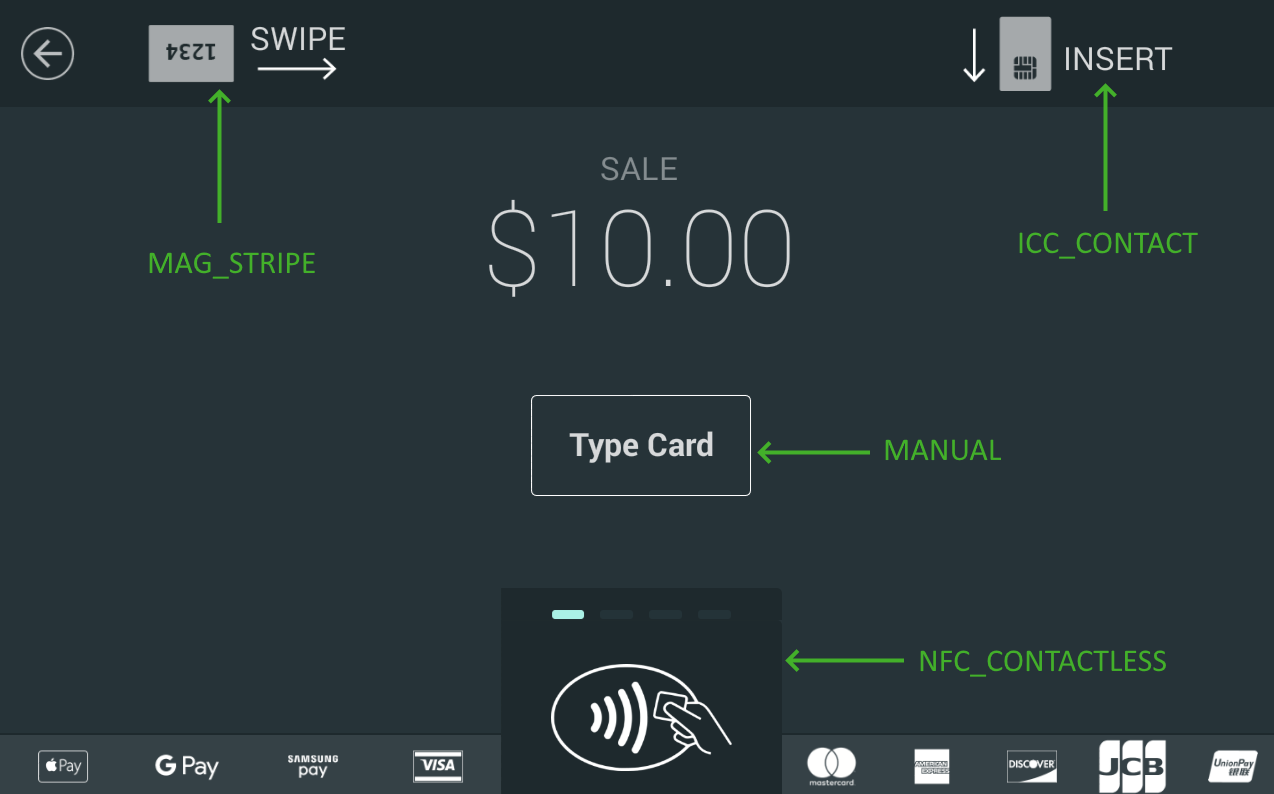
Card entry methods
Allow manual and all card entry methods:
- Add
CARD_ENTRY_METHOD_MANUAL
to allow manual card entry in addition to the default methods. - Set
CARD_ENTRY_METHODS.ALL
if you are using Remote Pay Cloud or Payment Connector.
Note
For Payment Card Industry Data Security Standard (PCI DSS) compliance, card information can only be entered on the Clover device; card information for the customer cannot be entered on your POS and then passed on to the device.
saleRequest.setCardEntryMethods(CloverConnector.CARD_ENTRY_METHOD_MANUAL |
CloverConnector.CARD_ENTRY_METHOD_MAG_STRIPE |
CloverConnector.CARD_ENTRY_METHOD_NFC_CONTACTLESS |
CloverConnector.CARD_ENTRY_METHOD_ICC_CONTACT);
saleRequest.setCardEntryMethods(CARD_ENTRY_METHODS.ALL);
Allow custom card entry method combination:
Set the values required by your merchants to allow a specific combination of entry methods. For example, to only allow EMV and contactless entry, and therefore disallow magstripe and manual entry, set the values as in the following example:
saleRequest.setCardEntryMethods(CardEntryMethods.CARD_ENTRY_METHOD_ICC_CONTACT|
CardEntryMethods.CARD_ENTRY_METHOD_NFC_CONTACTLESS);
Updated 6 months ago