Integrate with Clover Android SDK
North America
Europe
Latin America
Prerequisites
For this tutorial, you must have one item in your merchant's inventory. You can do this through the Inventory app.
Steps
- Use the Clover Android SDK to access merchant data. For this example, we will display information on one inventory item.
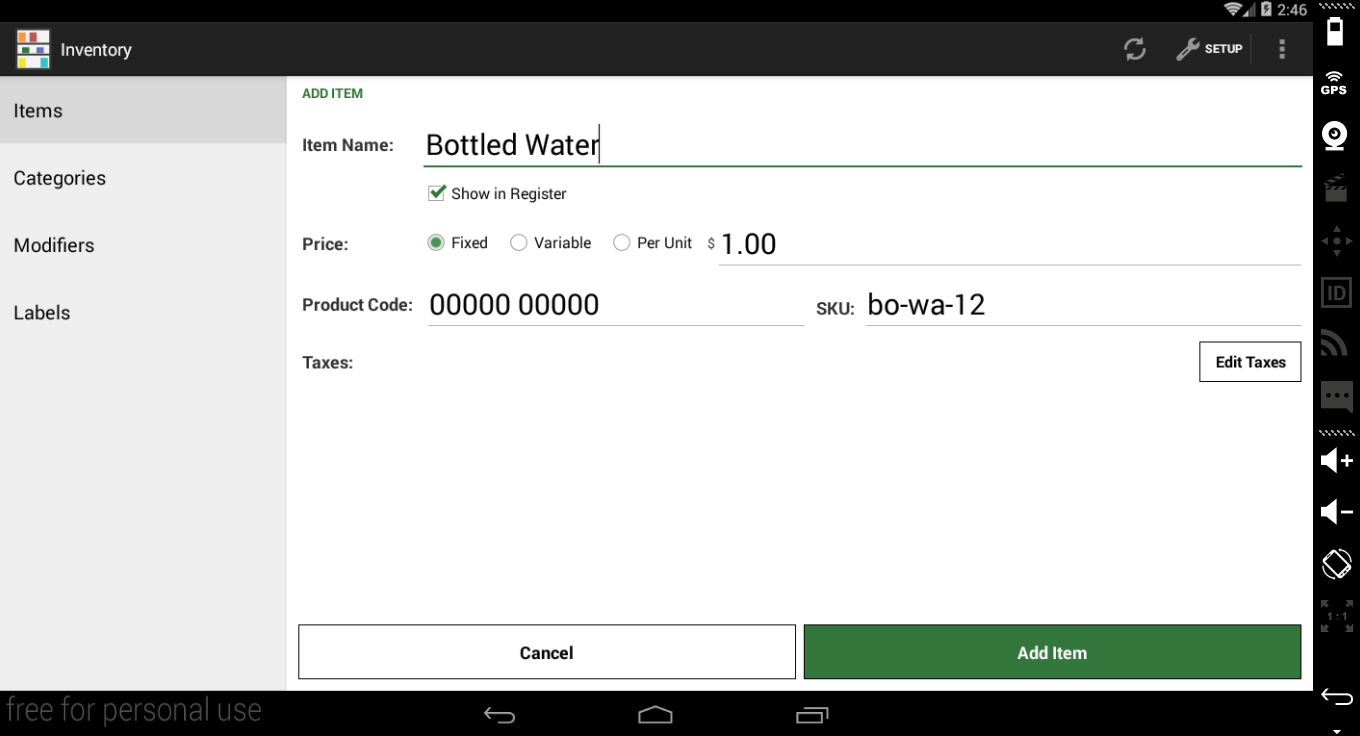
Inventory app: Items
- To integrate
clover-android-sdk
into your project, add a dependency to your Gradle-enabled project for either the latest release or a specific SDK version number, if you prefer.
Click a tab to see an example of each dependency:
dependencies {
implementation 'com.clover.sdk:clover-android-sdk:latest.release'
}
dependencies {
implementation 'com.clover.sdk:clover-android-sdk:VERSION_NUMBER'
}
Your gradle.build
file should display the dependency declaration in the dependencies section. In the following example, the version number dependency displays on line 25.
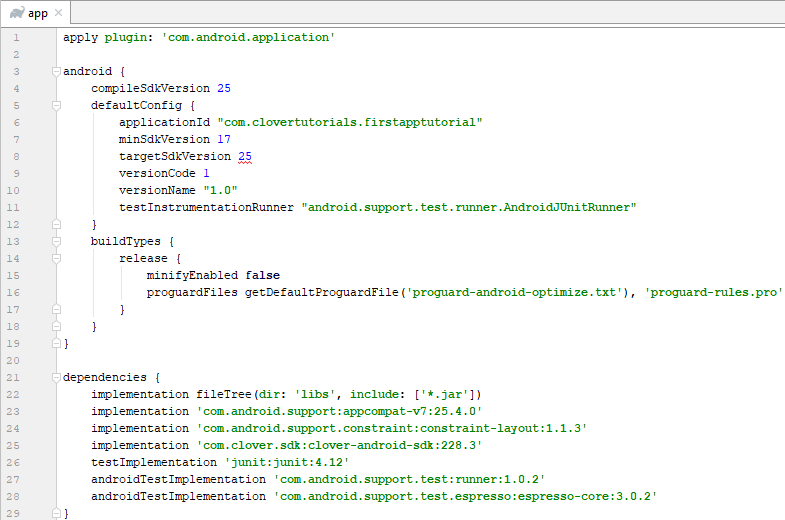
- Add an ID to the
textView
element to enable dynamic text insertion.
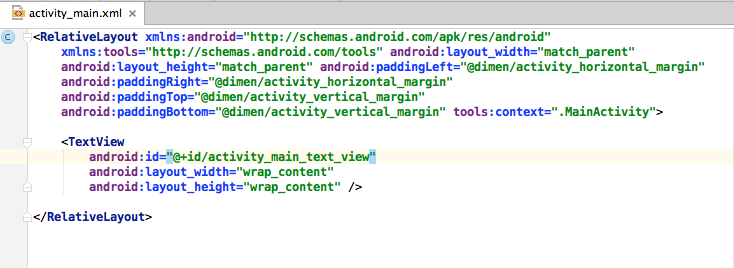
- Finally, you can replace your Activity with this code to pull out the merchant data:
/*
1) Replace <your.package.name.here> below with your package name.
*/
package com.your.package.name.here;
import android.accounts.Account;
import android.os.AsyncTask;
import android.os.RemoteException;
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import android.widget.TextView;
import com.clover.sdk.util.CloverAccount;
import com.clover.sdk.v1.BindingException;
import com.clover.sdk.v1.ClientException;
import com.clover.sdk.v1.ServiceException;
import com.clover.sdk.v3.inventory.InventoryConnector;
import com.clover.sdk.v3.inventory.Item;
import com.clover.sdk.v3.inventory.InventoryContract;
/*
2) To get or set any Clover data, go through Clover’s Connector classes,
such as InventoryConnector.
*/
public class MainActivity extends AppCompatActivity {
private Account mAccount;
private InventoryConnector mInventoryConnector;
private TextView mTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onResume() {
super.onResume();
mTextView = (TextView) findViewById(R.id.activity_main_text_view);
// Retrieve the Clover account
if (mAccount == null) {
mAccount = CloverAccount.getAccount(this);
if (mAccount == null) {
return;
}
}
// Connect InventoryConnector
connect();
// Get Item
new InventoryAsyncTask().execute();
}
/*
3) Disconnect from the Clover Connectors when you aren’t using them to
follow Android best practices and let the connection end naturally.
*/
@Override
protected void onDestroy() {
super.onDestroy();
disconnect();
}
private void connect() {
disconnect();
if (mAccount != null) {
mInventoryConnector = new InventoryConnector(this, mAccount, null);
mInventoryConnector.connect();
}
}
private void disconnect() {
if (mInventoryConnector != null) {
mInventoryConnector.disconnect();
mInventoryConnector = null;
}
}
/*
4) Use async tasks when working with Clover Connector classes to minimize
work on the main UI thread while fetching or changing data. See
https://docs.clover.com/clover-platform/docs/clover-development-
basics-android#section-async-tasks-and-executors.
*/
private class InventoryAsyncTask extends AsyncTask<Void, Void, Item> {
@Override
protected final Item doInBackground(Void... unused) {
String itemId = null;
try (Cursor cursor = getContentResolver().query(InventoryContract.Item.contentUriWithAccount(mAccount),
new String[] { InventoryContract.Item.UUID }, null, null, null)){
//Get inventory item
if (cursor != null && cursor.moveToFirst()) {
itemId = cursor.getString(0);
}
return itemId != null ? mInventoryConnector.getItem(itemId) : null;
} catch (RemoteException | ClientException | ServiceException | BindingException e) {
e.printStackTrace();
}
return null;
}
/*
5) Set the TextView to display the item’s name. For other methods that Item
objects have, see
https://clover.github.io/clover-android-sdk/com/clover/sdk/v3/inventory
/Item.html
*/
@Override
protected final void onPostExecute(Item item) {
if (item != null) {
mTextView.setText(item.getName());
}
}
}
}
- Sideload your app onto your device to see it in action. For more information, see Sideload an APK.
Updated 6 months ago