Tokenize a card
Use theTokenizeCardRequestIntentBuilder
to tokenize a card for Clover Ecommerce endpoints.
Prerequisites
Clover devices must have the Core Payments App (CPA) installed to use the card tokenization feature. CPA is installed in all Clover Gen 3 devices. If your device does not have CPA installed, contact Clover support to use this feature.
Build a tokenize request
You can build a tokenize card request with a few lines of code.
val context = this
val builder = TokenizeCardRequestIntentBuilder()
val intent = builder.build(context)
Context context = this;
TokenizeCardRequestIntentBuilder builder = new TokenizeCardRequestIntentBuilder();
Intent intent = builder.build(context);
CardOptions
The CardOptions
class provides a static method option to set the supported card entry methods.
Method | Description |
---|---|
Instance(cardEntryMethods : Set<CardEntryMethod>) | Set the cardEntryMethods flag to create a CardOptions instance. You can limit the card entry methods—for example, CardReaders(), Manual(), All(). |
Example—Set the card entry method to CardReaders
val builder = TokenizeCardRequestIntentBuilder()
val cardOptions = TokenizeCardRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.CardReaders())
val intent = builder.cardOptions(cardOptions).build(context)
TokenizeCardRequestIntentBuilder builder = new TokenizeCardRequestIntentBuilder();
TokenizeCardRequestIntentBuilder.CardOptions cardOptions = TokenizeCardRequestIntentBuilder.CardOptions.Instance(CardEntryMethod.CardReaders());
Intent intent = builder.cardOptions(cardOptions).build(context);
Additional fields
Field | Description |
---|---|
suppressConfirmation:Boolean? | Use to suppress—or skip—the confirmation pop-up that asks the customer if the merchant can store the customer's card information |
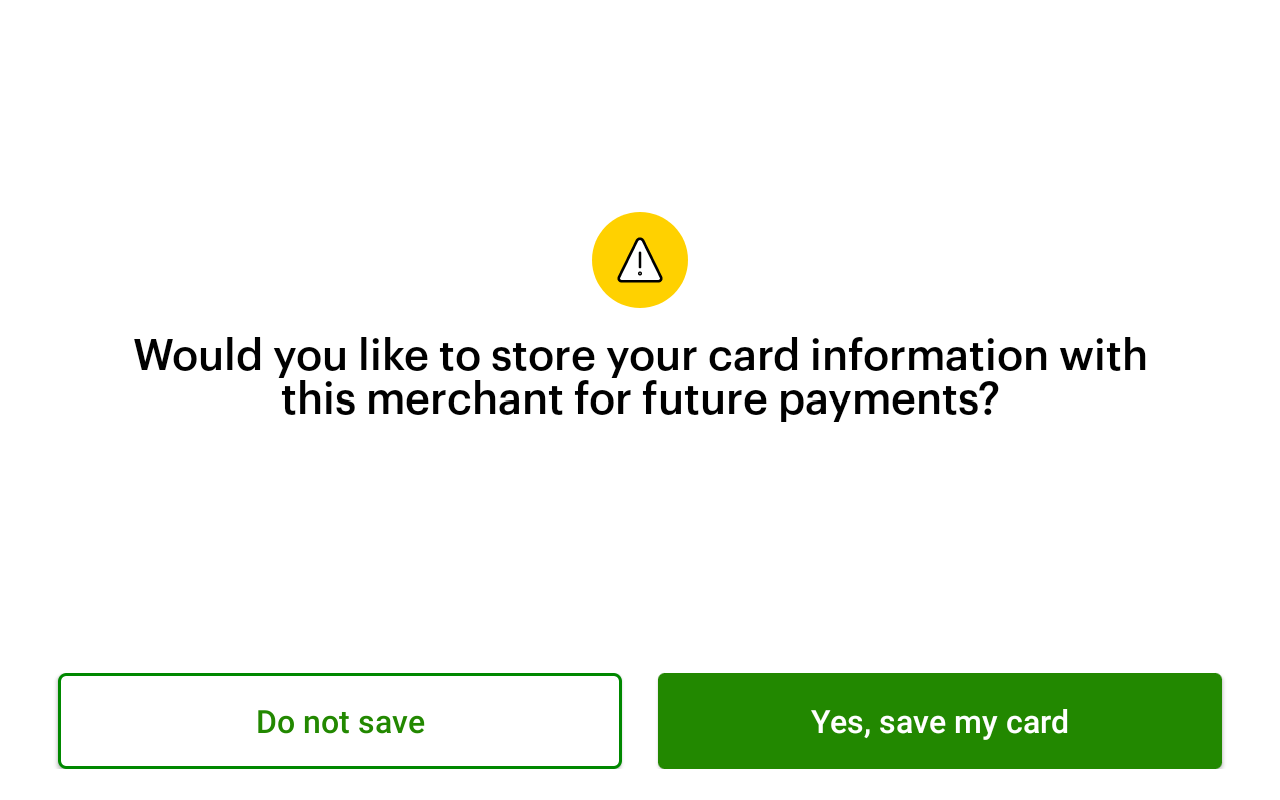
Token confirmation pop-up
Response details
- When the tokenize card flow completes, the response returns through
onActivityResult()
. - After a successful request, you can retrieve objects such as the token, token type, confirmation suppression status, and card.
- If the request fails, you can retrieve the failure message.
Response example
fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == TOKENIZE_CARD_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
val token = data.getStringExtra(Intents.EXTRA_TOKEN)
val tokenType = data.getStringExtra(Intents.EXTRA_TOKEN_TYPE)
val suppressConfirmation = data.getBooleanExtra(Intents.EXTRA_SUPPRESS_CONFIRMATION, false)
val card:Card = data.getParcelableExtra(Intents.EXTRA_CARD)
} else {
// tokenize failed, check for error
val failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE)
}
}
}
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == TOKENIZE_CARD_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
String token = data.getStringExtra(Intents.EXTRA_TOKEN);
String tokenType = data.getStringExtra(Intents.EXTRA_TOKEN_TYPE);
boolean suppressConfirmation = data.getBooleanExtra(Intents.EXTRA_SUPPRESS_CONFIRMATION, false);
Card card = data.getParcelableExtra(Intents.EXTRA_CARD);
} else {
// tokenize failed, check for error
String failureMessage = data.getStringExtra(Intents.EXTRA_FAILURE_MESSAGE);
}
}
}
Additional requirements for developers
If your application stores tokens to use in subsequent transactions, you are responsible for complying with card-on-file (COF) mandates, also called the Stored Credentials Transaction framework.
Clover collects a general consent agreement from the customer to proceed with either a card tokenization request and/or payment. Card-on-file rules require additional steps that merchants and software developers must take to reach full compliance. Merchants are still responsible to perform the following activities when saving customer cards (tokens) on file:
- Inform the account issuer that payment credentials are now stored on file. To do this either process an initial payment or complete a $0 account verification through the Clover REST Pay Display API.
- Disclose to cardholders how the credentials will be used.
- Notify cardholders when any changes are made to the terms of use.
For more information about safekeeping tokenized card data, see our blog Ecommerce Tokenization: Understanding Methods that Keep Card Data Safe.
Updated 8 months ago