Android—Device connection
Overview
To connect to a Clover Go reader, discover devices within Bluetooth (BLE) range and request a connection. During the connection process, the Clover Go software development kit (SDK) checks for valid reader and merchant configuration settings and updates firmware and encryption keys as needed.
Prerequisites
- See Overview of the Clover platform.
- Create a global developer account with a default test merchant account.
- Order a Clover Go reader Developer Kit (Dev Kit) and set it up.
- Use required Android SDK versions.
- Have BLUETOOTH_CONNECT permission in your AndroidManifest to request the devices list. Android uses the permission to request BLE access to your app's user.
Steps
1. Discover devices
Initiate device discovery with the Clover GoSDK.scanForReaders function. Receive results back with collectLatest on the flow function or using the GoSdkCallback implementation.
Methods
fun scanForReaders(): Flow<ReaderInfo>
fun scanForReaders(lifecycleOwner: LifecycleOwner, callback: GoSdkCallback<ReaderInfo>)
//lifecycleOwner: Class that contains Android Activity/Fragment Lifecycle information.
//callback: Callback interface to get ReaderInfo results or error
interface GoSdkCallback<ReaderInfo> {
fun onNext(result: ReaderInfo) //Gets called when a card reader is discovered
//result: Card reader object
fun onError(e: Throwable) //Gets called when there is an error while scanning for readers
//e: Throwable exception containing error information
}
Recommended implementation
Scanning for devices can take time. For example, the user might not have turned on their device when they first scan for it unless you remind them to.
- Implement a backing data store for the ListView or RecyclerView in which you want to present the list of readers.
- Call GoSdk.scanForReaders() in a coroutine scope or suspend function, add the discovered reader to your backing data source, and handle any errors returned in onScanError.
lifecycleScope.launch {
goSdk.scanForReaders()
.collectLatest { reader->
println("Scanned Reader: $reader")
recyclerViewAdapter.addDevice(reader)
}
.catch { error->
println("Error scanning for readers: $error")
}
}
2. Connect to a discovered device
- Call the Clover GoSDK.connectBluetoothReader method. Pass in the reader object you received from the discovery process.
- To receive the device connection status callbacks, call Clover GoSDK.observeCardReaderStatus.
- To subscribe to device connection status callbacks, call collectLatest on the flow function or use the GoSdkCallback implementation.
Methods
fun connectBluetoothReader(readerInfo: ReaderInfo)
//readerInfo: The specific `CardReader` to connect to
fun observeCardReaderStatus(): SharedFlow<CardReaderStatus>
fun observeCardReaderStatus(lifecycleOwner: LifecycleOwner, callback: GoSdkCallback<CardReaderStatus>)
//lifecycleOwner: Class that contains Android Activity/Fragment Lifecycle information.
//callback: Callback interface to get CardReaderStatus results or error
interface GoSdkCallback<CardReaderStatus> {
fun onNext(result: CardReaderStatus) //Gets called when the CardReaderStatus gets updated
//result: Card reader object
fun onError(e: Throwable) //Gets called when there is an error while scanning for readers
//e: Throwable exception containing error information
}
sealed class CardReaderStatus {
data class Disconnected(val readerInfo: ReaderInfo) : CardReaderStatus()
//readerInfo: CardReader object containing all available information
data class Deleted(val readerInfo: ReaderInfo) : CardReaderStatus()
//readerInfo: CardReader object containing all available information
data class Connected(val readerInfo: ReaderInfo) : CardReaderStatus()
//readerInfo: CardReader object containing all available information
object Connecting : CardReaderStatus()
object CheckingForUpdate : CardReaderStatus()
data class Ready(val readerInfo: ReaderInfo) : CardReaderStatus()
//readerInfo: CardReader object containing all available information
data class ResetInProgress(val readerType: ReaderInfo.ReaderType) : CardReaderStatus()
//readerType: The type of reader we're connecting to. One of: RP350, RP450 or C600
object UpdateComplete : CardReaderStatus()
data class BleFirmwareUpdateInProgress(val progress: Int) : CardReaderStatus()
//progress: Firmware Update percentage from 0-100
data class SpFirmwareUpdateInProgress(val progress: Int) : CardReaderStatus()
//progress: Firmware Update percentage from 0-100
object RkiInProgress : CardReaderStatus()
data class BatteryPercentChanged(val readerInfo: ReaderInfo) : CardReaderStatus()
//readerInfo: CardReader object containing all available information
fun isConnected(): Boolean = !(this is Disconnected || this is Deleted)
}
Connection flow
When you connect to a card reader for the first time, you may need to update firmware and encryption keys which can take a few minutes. Subsequent connections take a few seconds as the configuration is checked and verified as valid. It is important that you inform your users during this process that the device is connecting and undergoing needed updates. Use the status information to inform your users about the connection flow process. A typical connection flow status is shown below.
connectBluetoothReader > Connecting > Connected > CheckingForUpdate > ResetInProgress (optional) > BleFirmwareUpdateInProgress (optional) > SpFirmwareUpdateInProgress (optional) > UpdateComplete (optional) > RkiInProgress (optional) > Ready
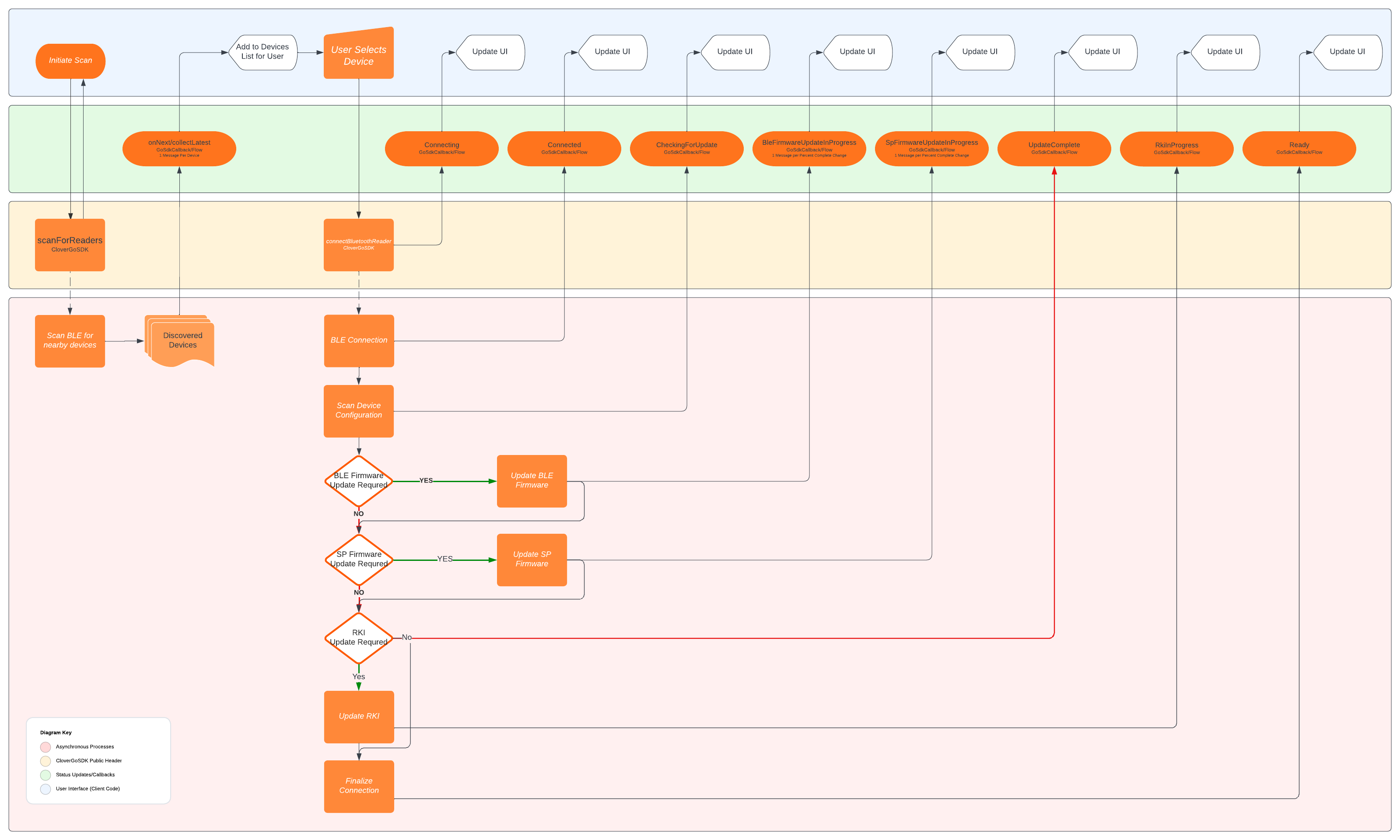
connectBluetoothReader
Your code calls this method to initiate the connection, passing in a reader object received during the discovery process. Depending on the use cases of your app, you may automatically connect to the last connected reader, or you may present your users with a list of readers to choose from. When you call this method, the Clover Go SDK:
- Establishes a BLE connection to the reader.
- Requests the reader's configuration from the reader.
- Inspects the configuration.
- Begins the firmware and encryption key update process.
NOTE
The update process involves network calls to Clover servers to look for software updates and is performed every time a reader connects, and so network conditions may delay the completion of this process.
HINT
Since this call initiates a BLE connection with the Clover reader, you can expect to navigate the specific OS BLE pairing process during this time. The OS asks if the user wants to connect to the specified device, but the SDK handles the pairing code part of the process without user input.
Status descriptions
Status | Description |
---|---|
Connected | Successful BLE connection with the device. Note: The CardReader object(s) may not be fully populated at this point. The battery status is not populated until the first Ready status and is not reliable at this point. |
ResetInProgress | Follows onConnected and signals the start of the update process. |
BleFirmwareUpdateInProgress | BLE firmware update is required. A series of updates on this status returns. Expect to receive one status update for every whole number change of the percent complete. If no BLE firmware updates are found to be required, this status does not occur. |
SpFirmwareUpdateInProgress | Secure Processor (SP) firmware update is required. A series of updates on this status returns. Expect to receive one status update for every whole number change of the percent complete. If no SP firmware updates are found to be required, this status does not occur. |
RkiInProgress | Encryption keys were updated. This process may take up to 1 minute to complete, during which no status updates occur. |
Ready | Clover Go reader is fully connected, configured, and ready to take a payment. The CardReader object passed in this callback is fully populated. Use it to update your user interface (UI). |
Updated about 1 month ago